diff --git a/examples/openapitool.ipynb b/examples/openapitool.ipynb
new file mode 100644
index 00000000..ab0e54b9
--- /dev/null
+++ b/examples/openapitool.ipynb
@@ -0,0 +1,547 @@
+{
+ "cells": [
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "HCnOg0poyvSo"
+ },
+ "source": [
+ "# ๐งช Invoking APIs with `OpenAPITool`\n",
+ "\n",
+ "\n",
+ "\n",
+ "Many APIs available on the Web provide an OpenAPI specification that describes their structure and syntax.\n",
+ "\n",
+ "[`OpenAPITool`](https://docs.haystack.deepset.ai/reference/openapi-api) is an experimental Haystack component that allows you to call an API using payloads generated from human instructions.\n",
+ "\n",
+ "Here's a brief overview of how it works:\n",
+ "- At initialization, it loads the OpenAPI specification from a URL or a file.\n",
+ "- At runtime:\n",
+ " - Converts human instructions into a suitable API payload using a Chat Language Model (LLM).\n",
+ " - Invokes the API.\n",
+ " - Returns the API response, wrapped in a Chat Message.\n",
+ "\n",
+ "Let's see this component in action..."
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "ruIf93lVLaO9"
+ },
+ "source": [
+ "## Setup"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "id": "XvsPuqZcaIvp"
+ },
+ "outputs": [],
+ "source": [
+ "! pip install haystack-ai jsonref"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "ew6t1MY1LkP7"
+ },
+ "source": [
+ "In this notebook, we will be using some APIs that require an API key. Let's set them as environment variables."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "id": "eOz5Ry4IaOub"
+ },
+ "outputs": [],
+ "source": [
+ "import os\n",
+ "\n",
+ "os.environ[\"OPENAI_API_KEY\"]=\"...\"\n",
+ "\n",
+ "# free API key: https://www.firecrawl.dev/\n",
+ "os.environ[\"FIRECRAWL_API_KEY\"]=\"...\"\n",
+ "\n",
+ "# free API key: https://serper.dev/\n",
+ "os.environ[\"SERPERDEV_API_KEY\"]=\"...\""
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "8NUEM_uVfpdi"
+ },
+ "source": [
+ "## Call an API without credentials\n",
+ "\n",
+ "In the first example, we use Open-Meteo, a Free Weather API that does not require authentication.\n",
+ "\n",
+ "We use `OPENAI` as LLM provider. Other supported providers are `ANTHROPIC` and `COHERE`."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "colab": {
+ "base_uri": "https://localhost:8080/"
+ },
+ "id": "MDy213SLXZQy",
+ "outputId": "4e9e1607-4212-4ce7-e409-ded548f83ab0"
+ },
+ "outputs": [
+ {
+ "data": {
+ "text/plain": [
+ "{'service_response': [ChatMessage(content='{\"latitude\": 37.763283, \"longitude\": -122.41286, \"generationtime_ms\": 0.07903575897216797, \"utc_offset_seconds\": 0, \"timezone\": \"GMT\", \"timezone_abbreviation\": \"GMT\", \"elevation\": 18.0, \"current_weather_units\": {\"time\": \"iso8601\", \"interval\": \"seconds\", \"temperature\": \"\\\\u00b0C\", \"windspeed\": \"km/h\", \"winddirection\": \"\\\\u00b0\", \"is_day\": \"\", \"weathercode\": \"wmo code\"}, \"current_weather\": {\"time\": \"2024-07-22T16:45\", \"interval\": 900, \"temperature\": 18.1, \"windspeed\": 3.4, \"winddirection\": 212, \"is_day\": 1, \"weathercode\": 1}}', role=, name=None, meta={})]}"
+ ]
+ },
+ "execution_count": 2,
+ "metadata": {},
+ "output_type": "execute_result"
+ }
+ ],
+ "source": [
+ "from haystack.dataclasses import ChatMessage\n",
+ "from haystack_experimental.components.tools.openapi import OpenAPITool, LLMProvider\n",
+ "from haystack.utils import Secret\n",
+ "\n",
+ "tool = OpenAPITool(generator_api=LLMProvider.OPENAI,\n",
+ " spec=\"https://raw.githubusercontent.com/open-meteo/open-meteo/main/openapi.yml\")\n",
+ "\n",
+ "tool.run(messages=[ChatMessage.from_user(\"Weather in San Francisco, US\")])"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "EQmvkszfgZoi"
+ },
+ "source": [
+ "## Incorporate `OpenAPITool` in a Pipeline\n",
+ "\n",
+ "Next, let's create a simple Pipeline where the service response is translated into a human-understandable format using the Language Model.\n",
+ "\n",
+ "We use an [`OutputAdapter`](https://docs.haystack.deepset.ai/docs/outputadapter) to create a list of Chat Messages for the LM."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "colab": {
+ "base_uri": "https://localhost:8080/"
+ },
+ "id": "xTRcny9ig-0t",
+ "outputId": "d5f21778-b4fc-4eb1-8e3a-dbc6f99f9b44"
+ },
+ "outputs": [
+ {
+ "data": {
+ "text/plain": [
+ "\n",
+ "๐ Components\n",
+ " - meteo: OpenAPITool\n",
+ " - prompt_adapter: OutputAdapter\n",
+ " - llm: OpenAIChatGenerator\n",
+ "๐ค๏ธ Connections\n",
+ " - meteo.service_response -> prompt_adapter.service_response (List[ChatMessage])\n",
+ " - prompt_adapter.output -> llm.messages (List[ChatMessage])"
+ ]
+ },
+ "execution_count": 3,
+ "metadata": {},
+ "output_type": "execute_result"
+ }
+ ],
+ "source": [
+ "from typing import List\n",
+ "from haystack import Pipeline\n",
+ "from haystack.components.converters import OutputAdapter\n",
+ "from haystack.components.generators.chat import OpenAIChatGenerator\n",
+ "\n",
+ "pipe = Pipeline()\n",
+ "pipe.add_component(\"meteo\", tool)\n",
+ "pipe.add_component(\"prompt_adapter\", OutputAdapter(\"{{user_message + service_response}}\", List[ChatMessage]))\n",
+ "pipe.add_component(\"llm\", OpenAIChatGenerator(generation_kwargs={\"max_tokens\": 1024}))\n",
+ "\n",
+ "pipe.connect(\"meteo\", \"prompt_adapter.service_response\")\n",
+ "pipe.connect(\"prompt_adapter\", \"llm.messages\")"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "id": "6yF2RyfQhAaV"
+ },
+ "outputs": [],
+ "source": [
+ "result = pipe.run(data={\"meteo\": {\"messages\": [ChatMessage.from_user(\"weather in San Francisco, US\")]},\n",
+ " \"prompt_adapter\": {\"user_message\": [ChatMessage.from_user(\"Explain the weather in San Francisco in a human understandable way\")]}})"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "colab": {
+ "base_uri": "https://localhost:8080/"
+ },
+ "id": "RJg3P-k2hY5M",
+ "outputId": "938ca3f1-6c57-4969-8d56-ed9cab9258af"
+ },
+ "outputs": [
+ {
+ "name": "stdout",
+ "output_type": "stream",
+ "text": [
+ "Currently in San Francisco, the weather can be described as mild with a temperature of 18.1ยฐC (64.6ยฐF). The wind is blowing at a speed of 3.4 km/h coming from the south-southwest direction. It is daytime in San Francisco at the moment.\n"
+ ]
+ }
+ ],
+ "source": [
+ "print(result[\"llm\"][\"replies\"][0].content)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "2r5h2f0ehfrb"
+ },
+ "source": [
+ "## Use an API with credentials in a Pipeline\n",
+ "\n",
+ "In this example, we use [Firecrawl](https://www.firecrawl.dev/): a project that scrape Web pages (and Web sites) and convert them into clean text. Firecrawl has an API that requires an API key.\n",
+ "\n",
+ "In the following Pipeline, we use Firecrawl to scrape a news article, which is then summarized using a Language Model."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "colab": {
+ "base_uri": "https://localhost:8080/"
+ },
+ "id": "Y64kS9RbatCQ",
+ "outputId": "1db016c0-981f-4252-ce67-50bb8aae264e"
+ },
+ "outputs": [
+ {
+ "data": {
+ "text/plain": [
+ "\n",
+ "๐ Components\n",
+ " - firecrawl: OpenAPITool\n",
+ " - prompt_adapter: OutputAdapter\n",
+ " - llm: OpenAIChatGenerator\n",
+ "๐ค๏ธ Connections\n",
+ " - firecrawl.service_response -> prompt_adapter.service_response (List[ChatMessage])\n",
+ " - prompt_adapter.output -> llm.messages (List[ChatMessage])"
+ ]
+ },
+ "execution_count": 6,
+ "metadata": {},
+ "output_type": "execute_result"
+ }
+ ],
+ "source": [
+ "pipe = Pipeline()\n",
+ "pipe.add_component(\"firecrawl\", OpenAPITool(generator_api=LLMProvider.OPENAI,\n",
+ " spec=\"https://raw.githubusercontent.com/mendableai/firecrawl/main/apps/api/openapi.json\",\n",
+ " credentials=Secret.from_env_var(\"FIRECRAWL_API_KEY\")))\n",
+ "pipe.add_component(\"prompt_adapter\", OutputAdapter(\"{{user_message + service_response}}\", List[ChatMessage]))\n",
+ "pipe.add_component(\"llm\", OpenAIChatGenerator(generation_kwargs={\"max_tokens\": 1024}))\n",
+ "\n",
+ "pipe.connect(\"firecrawl\", \"prompt_adapter.service_response\")\n",
+ "pipe.connect(\"prompt_adapter\", \"llm.messages\")"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "id": "LSj6l6_6bRjT"
+ },
+ "outputs": [],
+ "source": [
+ "user_prompt = \"Given the article below, list the most important facts in a bulleted list. Do not include repetitions. Max 5 points.\"\n",
+ "\n",
+ "result = pipe.run(data={\"firecrawl\": {\"messages\": [ChatMessage.from_user(\"Scrape https://lite.cnn.com/2024/07/18/style/rome-ancient-papal-palace/index.html\")]},\n",
+ " \"prompt_adapter\": {\"user_message\": [ChatMessage.from_user(user_prompt)]}})"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "colab": {
+ "base_uri": "https://localhost:8080/"
+ },
+ "id": "c_TJ8R2VbWMM",
+ "outputId": "ac0128f3-a2c0-413b-8052-24f6730c6e4f"
+ },
+ "outputs": [
+ {
+ "name": "stdout",
+ "output_type": "stream",
+ "text": [
+ "- Remains of a medieval palace believed to be where popes lived before the Vatican have been excavated in Rome.\n",
+ "- The site is located near the Archbasilica of St John Lateran in Rome.\n",
+ "- The building's initial structure dates back to Emperor Constantine in the 4th century and was expanded between the 9th and 13th centuries.\n",
+ "- The papacy resided in the palace until 1305 when it temporarily moved to Avignon in France.\n",
+ "- The discovery was made ahead of renovations for the 2025 Catholic Holy Year, expected to attract over 30 million pilgrims and tourists to Rome.\n"
+ ]
+ }
+ ],
+ "source": [
+ "print(result[\"llm\"][\"replies\"][0].content)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "Wv6weSs3iQ40"
+ },
+ "source": [
+ "## Create a Pipeline with multiple `OpenAPITool` components\n",
+ "\n",
+ "In this example, we show a Pipeline where multiple alternative APIs can be invoked depending on the user query. In particular, a Google Search (via Serper.dev) can be performed or a single page can be scraped using Firecrawl.\n",
+ "\n",
+ "โ ๏ธ The approach shown is just one way to achieve this using [conditional routing](https://docs.haystack.deepset.ai/docs/conditionalrouter). We are currently experimenting with tool support in Haystack, and there may be simpler ways to achieve the same result in the future."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "id": "73ypFwhtKbH9"
+ },
+ "outputs": [],
+ "source": [
+ "import json\n",
+ "\n",
+ "decision_prompt_template = \"\"\"\n",
+ "You are a virtual assistant, equipped with the following tools:\n",
+ "\n",
+ "- `{\"tool_name\": \"search_web\", \"tool_description\": \"Access to Google search, use this tool whenever information on recents events is needed\"}`\n",
+ "- `{\"tool_name\": \"scrape_page\", \"tool_description\": \"Use this tool to scrape and crawl web pages\"}`\n",
+ "\n",
+ "Select the most appropriate tool to resolve the user's query. Respond in JSON format, specifying the user request and the chosen tool for the response.\n",
+ "If you can't match user query to an above listed tools, respond with `none`.\n",
+ "\n",
+ "\n",
+ "######\n",
+ "Here are some examples:\n",
+ "\n",
+ "```json\n",
+ "{\n",
+ " \"query\": \"Why did Elon Musk recently sue OpenAI?\",\n",
+ " \"response\": \"search_web\"\n",
+ "}\n",
+ "{\n",
+ " \"query\": \"What is on the front-page of hackernews today?\",\n",
+ " \"response\": \"scrape_page\"\n",
+ "}\n",
+ "{\n",
+ " \"query\": \"Tell me about Berlin\",\n",
+ " \"response\": \"none\"\n",
+ "}\n",
+ "```\n",
+ "\n",
+ "Choose the best tool (or none) for each user request, considering the current context of the conversation specified above.\n",
+ "\n",
+ "{\"query\": {{query}}, \"response\": }\n",
+ "\"\"\"\n",
+ "\n",
+ "def get_tool_name(replies):\n",
+ " try:\n",
+ " tool_name = json.loads(replies)[\"response\"]\n",
+ " return tool_name\n",
+ " except:\n",
+ " return \"error\"\n",
+ "\n",
+ "def create_chat_message(query):\n",
+ " return [ChatMessage.from_user(query)]\n",
+ "\n",
+ "\n",
+ "routes = [\n",
+ " {\n",
+ " \"condition\": \"{{replies[0] | get_tool_name == 'search_web'}}\",\n",
+ " \"output\": \"{{query | create_chat_message}}\",\n",
+ " \"output_name\": \"search_web\",\n",
+ " \"output_type\": List[ChatMessage],\n",
+ " },\n",
+ " {\n",
+ " \"condition\": \"{{replies[0] | get_tool_name == 'scrape_page'}}\",\n",
+ " \"output\": \"{{query | create_chat_message}}\",\n",
+ " \"output_name\": \"scrape_page\",\n",
+ " \"output_type\": List[ChatMessage],\n",
+ " },\n",
+ " {\n",
+ " \"condition\": \"{{replies[0] | get_tool_name == 'none'}}\",\n",
+ " \"output\": \"{{replies[0]}}\",\n",
+ " \"output_name\": \"no_tools\",\n",
+ " \"output_type\": str,\n",
+ " },\n",
+ " {\n",
+ " \"condition\": \"{{replies[0] | get_tool_name == 'error'}}\",\n",
+ " \"output\": \"{{replies[0]}}\",\n",
+ " \"output_name\": \"error\",\n",
+ " \"output_type\": str,\n",
+ " },\n",
+ "]"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "colab": {
+ "base_uri": "https://localhost:8080/"
+ },
+ "id": "0BCqEIsmNXJx",
+ "outputId": "cc3bcfa7-56b1-4f41-d38f-8b4f4dbcece9"
+ },
+ "outputs": [
+ {
+ "data": {
+ "text/plain": [
+ "\n",
+ "๐ Components\n",
+ " - prompt_builder: PromptBuilder\n",
+ " - llm: OpenAIGenerator\n",
+ " - router: ConditionalRouter\n",
+ " - search_web_tool: OpenAPITool\n",
+ " - scrape_page_tool: OpenAPITool\n",
+ "๐ค๏ธ Connections\n",
+ " - prompt_builder.prompt -> llm.prompt (str)\n",
+ " - llm.replies -> router.replies (List[str])\n",
+ " - router.search_web -> search_web_tool.messages (List[ChatMessage])\n",
+ " - router.scrape_page -> scrape_page_tool.messages (List[ChatMessage])"
+ ]
+ },
+ "execution_count": 10,
+ "metadata": {},
+ "output_type": "execute_result"
+ }
+ ],
+ "source": [
+ "from haystack.components.builders.prompt_builder import PromptBuilder\n",
+ "from haystack.components.routers import ConditionalRouter\n",
+ "from haystack.components.generators import OpenAIGenerator\n",
+ "\n",
+ "search_web_tool = OpenAPITool(generator_api=LLMProvider.OPENAI,\n",
+ " spec=\"https://bit.ly/serper_dev_spec_yaml\",\n",
+ " credentials=Secret.from_env_var(\"SERPERDEV_API_KEY\"))\n",
+ "\n",
+ "scrape_page_tool = OpenAPITool(generator_api=LLMProvider.OPENAI,\n",
+ " spec=\"https://raw.githubusercontent.com/mendableai/firecrawl/main/apps/api/openapi.json\",\n",
+ " credentials=Secret.from_env_var(\"FIRECRAWL_API_KEY\"))\n",
+ "\n",
+ "pipe = Pipeline()\n",
+ "pipe.add_component(\"prompt_builder\", PromptBuilder(template=decision_prompt_template))\n",
+ "pipe.add_component(\"llm\", OpenAIGenerator())\n",
+ "pipe.add_component(\"router\", ConditionalRouter(routes, custom_filters={\"get_tool_name\": get_tool_name,\n",
+ " \"create_chat_message\": create_chat_message}))\n",
+ "pipe.add_component(\"search_web_tool\", search_web_tool)\n",
+ "pipe.add_component(\"scrape_page_tool\", scrape_page_tool)\n",
+ "\n",
+ "pipe.connect(\"prompt_builder\", \"llm\")\n",
+ "pipe.connect(\"llm.replies\", \"router.replies\")\n",
+ "pipe.connect(\"router.search_web\", \"search_web_tool\")\n",
+ "pipe.connect(\"router.scrape_page\", \"scrape_page_tool\")"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "colab": {
+ "base_uri": "https://localhost:8080/"
+ },
+ "id": "93qfiQQqjNEy",
+ "outputId": "aefa654a-a8f9-43ae-a4d8-7fdc46d7910a"
+ },
+ "outputs": [
+ {
+ "data": {
+ "text/plain": [
+ "{'llm': {'meta': [{'model': 'gpt-3.5-turbo-0125',\n",
+ " 'index': 0,\n",
+ " 'finish_reason': 'stop',\n",
+ " 'usage': {'completion_tokens': 23,\n",
+ " 'prompt_tokens': 248,\n",
+ " 'total_tokens': 271}}]},\n",
+ " 'search_web_tool': {'service_response': [ChatMessage(content='{\"searchParameters\": {\"q\": \"UEFA European Football Championship winner\", \"type\": \"search\", \"engine\": \"google\"}, \"answerBox\": {\"title\": \"Spain national football teamUEFA European Championship / Latest Champion\", \"answer\": \"Spain national football team\"}, \"organic\": [{\"title\": \"UEFA European Championship - Wikipedia\", \"link\": \"https://en.wikipedia.org/wiki/UEFA_European_Championship\", \"snippet\": \"The most recent championship, held in Germany in 2024, was won by Spain, who lifted a record fourth European title after beating England 2\\\\u20131 in the final at the ...\", \"sitelinks\": [{\"title\": \"Finals\", \"link\": \"https://en.wikipedia.org/wiki/List_of_UEFA_European_Championship_finals\"}, {\"title\": \"European Championship in\", \"link\": \"https://en.wikipedia.org/wiki/European_Championship_in_football\"}, {\"title\": \"UEFA Women\\'s Championship\", \"link\": \"https://en.wikipedia.org/wiki/UEFA_Women%27s_Championship\"}, {\"title\": \"Euro 2024\", \"link\": \"https://en.wikipedia.org/wiki/UEFA_Euro_2024\"}], \"position\": 1}, {\"title\": \"Winners List of the UEFA European Championship - The Euros\", \"link\": \"https://www.topendsports.com/events/soccer/uefa-euros/winners.htm\", \"snippet\": \"Ten different countries have won the tournament: Spain have won four times, Germany has three titles, France and Italy with two titles while Portugal, ...\", \"position\": 2}, {\"title\": \"List of UEFA European Championship finals - Wikipedia\", \"link\": \"https://en.wikipedia.org/wiki/List_of_UEFA_European_Championship_finals\", \"snippet\": \"The winners of the first ever final, held in Paris in 1960, were the Soviet Union, who defeated Yugoslavia 2\\\\u20131 after extra time, while in the latest one, hosted ...\", \"sitelinks\": [{\"title\": \"History\", \"link\": \"https://en.wikipedia.org/wiki/List_of_UEFA_European_Championship_finals#History\"}, {\"title\": \"List of finals\", \"link\": \"https://en.wikipedia.org/wiki/List_of_UEFA_European_Championship_finals#List_of_finals\"}, {\"title\": \"Results by nation\", \"link\": \"https://en.wikipedia.org/wiki/List_of_UEFA_European_Championship_finals#Results_by_nation\"}], \"position\": 3}, {\"title\": \"UEFA Euro Winners List from 1960 to today - Marca.com\", \"link\": \"https://www.marca.com/en/football/uefa-euro/winners.html\", \"snippet\": \"Check the updated ranking of all the winners of the UEFA Euro Cup year by year. Record of European Championships throughout history in Marca English.\", \"sitelinks\": [{\"title\": \"Euro 2024 Live Scores\", \"link\": \"https://www.marca.com/en/scores/football/uefa-euro.html\"}, {\"title\": \"Euro 2024 Schedule\", \"link\": \"https://www.marca.com/en/football/uefa-euro/schedule.html\"}, {\"title\": \"Euro Stadiums Germany 2024\", \"link\": \"https://www.marca.com/en/football/uefa-euro/stadiums.html\"}], \"position\": 4}, {\"title\": \"Most titles | History | UEFA EURO\", \"link\": \"https://www.uefa.com/uefaeuro/history/winners/\", \"snippet\": \"View the official UEFA EURO winners list at UEFA.com. Find out which teams have lifted the most trophies since the competition began.\", \"sitelinks\": [{\"title\": \"Portugal 1-0 France\", \"link\": \"https://www.uefa.com/uefaeuro/match/2017907--portugal-vs-france/\"}, {\"title\": \"2012: Spain 4-0 Italy\", \"link\": \"https://www.uefa.com/uefaeuro/match/2003351--spain-vs-italy/\"}, {\"title\": \"West Germany vs USSR\", \"link\": \"https://www.uefa.com/uefaeuro/match/3838--west-germany-vs-ussr/\"}, {\"title\": \"Spain (1964)\", \"link\": \"https://www.uefa.com/uefaeuro/match/3996--spain-vs-ussr/\"}], \"position\": 5}, {\"title\": \"European Championship | History, Winners, & Facts | Britannica\", \"link\": \"https://www.britannica.com/sports/European-Championship\", \"snippet\": \"... football tournaments. Learn more about the European Championship, including its winners ... Also known as: Euro, European Nation\\'s Cup, UEFA European Championship.\", \"position\": 6}, {\"title\": \"UEFA European Championship News, Stats, Scores - ESPN\", \"link\": \"https://www.espn.com/soccer/league/_/name/uefa.euro\", \"snippet\": \"Follow all the latest UEFA European Championship football news, fixtures, stats, and more on ESPN.\", \"position\": 7}, {\"title\": \"UEFA Euro winners: Know the champions - full list\", \"link\": \"https://olympics.com/en/news/uefa-european-championships-euro-winners-list-champions\", \"snippet\": \"Know all the UEFA European Championship winners. The Soviet Union won the first title in 1960 while Spain won the UEFA Euro 2024.\", \"date\": \"Jul 11, 2021\", \"position\": 8}, {\"title\": \"History | UEFA EURO\", \"link\": \"https://www.uefa.com/uefaeuro/history/\", \"snippet\": \"Official UEFA EURO history. Season-by-season guide, extensive all-time stats, plus video highlights of every final to date.\", \"sitelinks\": [{\"title\": \"Most titles\", \"link\": \"https://www.uefa.com/uefaeuro/history/winners/\"}, {\"title\": \"UEFA European...\", \"link\": \"https://www.uefa.com/uefaeuro/history/news/0253-0d81c56ff408-45bf000cd5b6-1000--uefa-european-championship-roll-of-honour/\"}, {\"title\": \"2020\", \"link\": \"https://www.uefa.com/uefaeuro/history/seasons/2020/\"}, {\"title\": \"All-time stats\", \"link\": \"https://www.uefa.com/uefaeuro/history/rankings/\"}], \"position\": 9}, {\"title\": \"UEFA EURO all-time winners 2024 - Statista\", \"link\": \"https://www.statista.com/statistics/378217/uefa-euro-titles-winners-and-finalists/\", \"snippet\": \"La Roja most recently won the competition in 2024, defeating England 2-1 in the EURO 2024 final. Read more. Countries with the most men\\'s UEFA ...\", \"date\": \"6 days ago\", \"position\": 10}], \"peopleAlsoAsk\": [{\"question\": \"Who won UEFA European Championship?\", \"snippet\": \"Spain national football team\\\\nUEFA European Championship / Latest Champion\", \"title\": \"\"}, {\"question\": \"Who is the winner of European Champions League?\", \"snippet\": \"Real Madrid CF\\\\nUEFA Champions League / Latest Champion\", \"title\": \"\"}, {\"question\": \"How many countries have won the European football Championship?\", \"snippet\": \"Final tournament Map of countries\\' best results. 10 countries have won, counting Germany and West Germany as one.\", \"title\": \"UEFA European Championship - Wikipedia\", \"link\": \"https://en.wikipedia.org/wiki/UEFA_European_Championship\"}, {\"question\": \"Who is the current women\\'s European champion?\", \"snippet\": \"The competition is the women\\'s equivalent of the UEFA European Championship. The reigning champions are England, who won their home tournament in 2022. The most successful nation in the history of the tournament is Germany, with eight titles.\", \"title\": \"UEFA Women\\'s Championship - Wikipedia\", \"link\": \"https://en.wikipedia.org/wiki/UEFA_Women%27s_Championship\"}], \"relatedSearches\": [{\"query\": \"UEFA Euro 2020 Final\"}, {\"query\": \"Uefa european football championship winner list\"}, {\"query\": \"Most euro cup winners list\"}, {\"query\": \"Last Euro Cup winners\"}, {\"query\": \"Uefa european football championship winner 2021\"}, {\"query\": \"Euro Cup winners list men\\'s\"}, {\"query\": \"Euro winners list since 2000\"}, {\"query\": \"Euro Cup winners list 2024\"}, {\"query\": \"Next Euro Cup 2024\"}]}', role=, name=None, meta={})]}}"
+ ]
+ },
+ "execution_count": 12,
+ "metadata": {},
+ "output_type": "execute_result"
+ }
+ ],
+ "source": [
+ "query = \"Who won the UEFA European Football Championship?\"\n",
+ "\n",
+ "pipe.run({\"prompt_builder\": {\"query\": query}, \"router\": {\"query\": query}})"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "colab": {
+ "base_uri": "https://localhost:8080/"
+ },
+ "id": "T2nC2yXGipRS",
+ "outputId": "c347781a-839a-4d04-8f66-d03101701a8e"
+ },
+ "outputs": [
+ {
+ "data": {
+ "text/plain": [
+ "{'llm': {'meta': [{'model': 'gpt-3.5-turbo-0125',\n",
+ " 'index': 0,\n",
+ " 'finish_reason': 'stop',\n",
+ " 'usage': {'completion_tokens': 26,\n",
+ " 'prompt_tokens': 250,\n",
+ " 'total_tokens': 276}}]},\n",
+ " 'scrape_page_tool': {'service_response': [ChatMessage(content='{\"success\": true, \"data\": {\"content\": \"\\\\n\\\\n[British Broadcasting Corporation](/)\\\\n\\\\n[Watch Live](/watch-live-news)\\\\n\\\\nRegisterSign In\\\\n\\\\n* [Home](/)\\\\n \\\\n* [News](/news)\\\\n \\\\n* [Sport](/sport)\\\\n \\\\n* [Business](/business)\\\\n \\\\n* [Innovation](/innovation)\\\\n \\\\n* [Culture](/culture)\\\\n \\\\n* [Travel](/travel)\\\\n \\\\n* [Earth](/future-planet)\\\\n \\\\n* [Video](/video)\\\\n \\\\n* [Live](/live)\\\\n \\\\n\\\\nRegisterSign In\\\\n\\\\n[Home](/)\\\\n\\\\nNews\\\\n\\\\n[Sport](/sport)\\\\n\\\\nBusiness\\\\n\\\\nInnovation\\\\n\\\\nCulture\\\\n\\\\nTravel\\\\n\\\\nEarth\\\\n\\\\n[Video](/video)\\\\n\\\\nLive\\\\n\\\\n[Audio](https://www.bbc.co.uk/sounds)\\\\n\\\\n[Weather](https://www.bbc.com/weather)\\\\n\\\\n[Newsletters](https://www.bbc.com/newsletters)\\\\n\\\\n[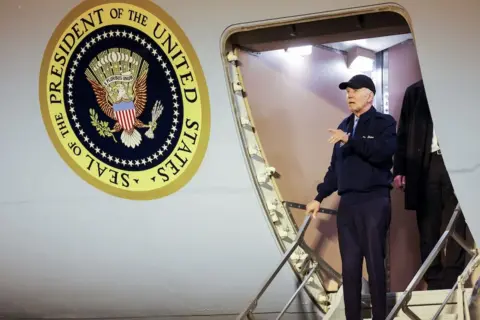\\\\\\\\\\\\n\\\\\\\\\\\\nThe last days of the Biden campaign \\\\u2013 BBC correspondent\\\\u2019s account\\\\\\\\\\\\n-----------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nBBC correspondent Tom Bateman takes us behind the scenes in the final days of Biden\\'s campaign.\\\\\\\\\\\\n\\\\\\\\\\\\n3 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/c250zqgrpqgo)\\\\n\\\\n[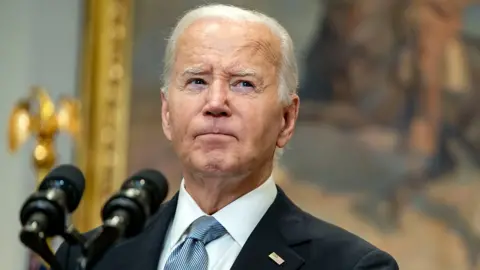\\\\\\\\\\\\n\\\\\\\\\\\\nIsolating at a beach house, Biden gave aides one minute notice of exit\\\\\\\\\\\\n----------------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nMost of the president\\'s senior aides found out about his decision to exit the race minutes before he publicly announced it.\\\\\\\\\\\\n\\\\\\\\\\\\n15 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/c3gdzmdje5xo)\\\\n\\\\n[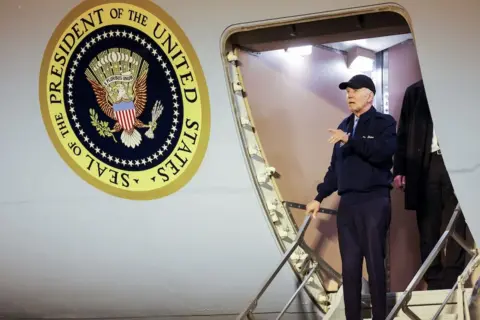\\\\\\\\\\\\n\\\\\\\\\\\\nThe last days of the Biden campaign \\\\u2013 BBC correspondent\\\\u2019s account\\\\\\\\\\\\n-----------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nBBC correspondent Tom Bateman takes us behind the scenes in the final days of Biden\\'s campaign.\\\\\\\\\\\\n\\\\\\\\\\\\n3 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/c250zqgrpqgo)\\\\n\\\\n[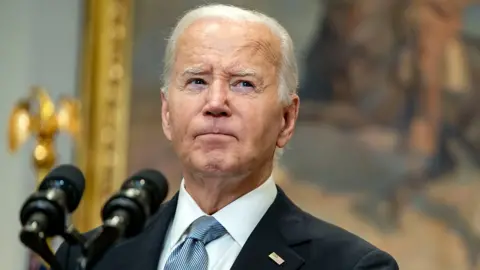\\\\\\\\\\\\n\\\\\\\\\\\\nIsolating at a beach house, Biden gave aides one minute notice of exit\\\\\\\\\\\\n----------------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nMost of the president\\'s senior aides found out about his decision to exit the race minutes before he publicly announced it.\\\\\\\\\\\\n\\\\\\\\\\\\n15 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/c3gdzmdje5xo)\\\\n\\\\n[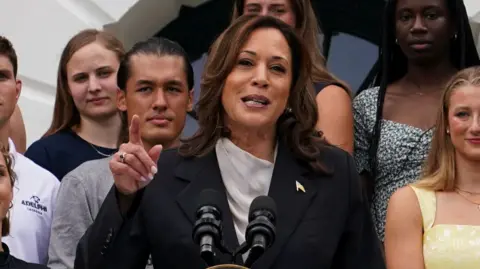\\\\\\\\\\\\n\\\\\\\\\\\\nLIVE\\\\\\\\\\\\n\\\\\\\\\\\\nKamala Harris speaks for first time since Biden left race - as endorsements mount\\\\\\\\\\\\n---------------------------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThe US vice-president appears at the White House for a pre-scheduled event - as key Democrats line up to back her candidacy.](https://www.bbc.com/news/live/cv2gryx1yx1t)\\\\n\\\\n* * *\\\\n\\\\n[What Biden quitting means for Harris, the Democrats and Trump\\\\\\\\\\\\n-------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nPresident Biden has upended the 2024 White House race for the Democrats. Here is what it means for Kamala Harris, his party and Trump.\\\\\\\\\\\\n\\\\\\\\\\\\n19 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/cpwd8yzw45qo)\\\\n\\\\n[Who could be Kamala Harris\\'s running mate?\\\\\\\\\\\\n------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nIt\\\\u2019s not a done deal but some potential rivals have quickly thrown their support behind her.\\\\\\\\\\\\n\\\\\\\\\\\\n10 mins ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/c80ekdwk9zro)\\\\n\\\\n[LIVE\\\\\\\\\\\\n\\\\\\\\\\\\nTensions flare as Congress presses Secret Service boss on \\'failed\\' Trump rally security\\\\\\\\\\\\n---------------------------------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nMembers of both parties have called for Kimberly Cheatle to resign in a House committee hearing that is seeking answers over security failures at the rally on 13 July.](https://www.bbc.com/news/live/c724wqpy4qnt)\\\\n\\\\n[The president\\'s protectors are hardly noticeable - until things go wrong\\\\\\\\\\\\n------------------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThe attempted assassination of Donald Trump has raised questions about the Secret Service\\'s record.\\\\\\\\\\\\n\\\\\\\\\\\\n6 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/c16j896003xo)\\\\n\\\\n* * *\\\\n\\\\nOnly from the BBC\\\\n-----------------\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\nWhy you are probably sitting for too long\\\\\\\\\\\\n-----------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nSitting down is ingrained in most peoples\\' days. But staying sedentary for too long can increase the risk of serious health conditions like cardiovascular disease and type 2 diabetes.\\\\\\\\\\\\n\\\\\\\\\\\\n4 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nFuture](/future/article/20240722-why-you-are-probably-sitting-down-for-too-long)\\\\n\\\\n[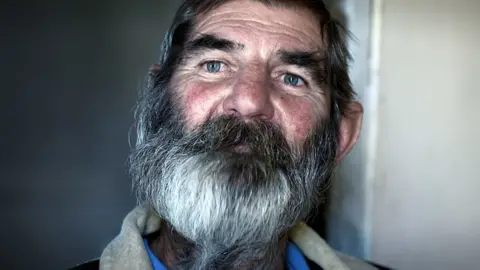\\\\\\\\\\\\n\\\\\\\\\\\\nMass killer who \\\\u2018hunted\\\\u2019 black people says police encouraged him\\\\\\\\\\\\n----------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nEx-security guard, Louis van Schoor, killed dozens in South Africa but was only jailed for seven murders.\\\\\\\\\\\\n\\\\\\\\\\\\n17 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nAfrica](/news/articles/c51yqdy3q61o)\\\\n\\\\n* * *\\\\n\\\\n[More news\\\\\\\\\\\\n---------](https://www.bbc.com/news)\\\\n\\\\u00a0\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\nUAE jails 57 Bangladeshis over protests against own government\\\\\\\\\\\\n--------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThree defendants were sentenced to life after being convicted of \\\\\"inciting riots\\\\\" in the Gulf state.\\\\\\\\\\\\n\\\\\\\\\\\\n5 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nWorld](/news/articles/crgk8gnpg0zo)\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\nIsrael orders evacuation of part of Gaza humanitarian zone\\\\\\\\\\\\n----------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nStrikes are reported near Khan Younis, after people are told to leave eastern areas in the zone.\\\\\\\\\\\\n\\\\\\\\\\\\n2 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nMiddle East](/news/articles/cgerz8we1vgo)\\\\n\\\\n[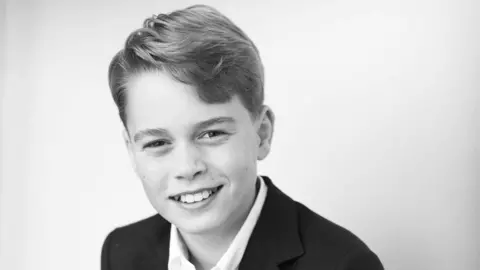\\\\\\\\\\\\n\\\\\\\\\\\\nNew Prince George photo released on 11th birthday\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nKensington Palace has posted the image, taken by his mother, Catherine, Princess of Wales.\\\\\\\\\\\\n\\\\\\\\\\\\n7 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUK](/news/articles/cw4yxd3dw7qo)\\\\n\\\\n[Piastri wins in Hungary after Norris team orders row\\\\\\\\\\\\n----------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nOscar Piastri takes his maiden grand prix victory ahead of McLaren team-mate Lando Norris in a dramatic race in Hungary.\\\\\\\\\\\\n\\\\\\\\\\\\n1 day ago\\\\\\\\\\\\n\\\\\\\\\\\\nFormula 1](/sport/formula1/articles/cg3jzy3e8q1o)\\\\n\\\\n[India alert after boy dies from Nipah virus in Kerala\\\\\\\\\\\\n-----------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThe virus is transmitted from animals such as pigs and fruit bats to humans.\\\\\\\\\\\\n\\\\\\\\\\\\n6 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nAsia](/news/articles/cj50d7e9vp6o)\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\nIsrael orders evacuation of part of Gaza humanitarian zone\\\\\\\\\\\\n----------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nStrikes are reported near Khan Younis, after people are told to leave eastern areas in the zone.\\\\\\\\\\\\n\\\\\\\\\\\\n2 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nMiddle East](/news/articles/cgerz8we1vgo)\\\\n\\\\n[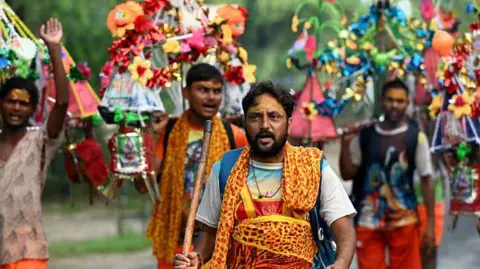\\\\\\\\\\\\n\\\\\\\\\\\\nIndia court blocks order for eateries to display owners\\' names\\\\\\\\\\\\n--------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nCritics say ordering restaurants to prominently display names of owners is discriminatory towards Muslims.\\\\\\\\\\\\n\\\\\\\\\\\\n6 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nAsia](/news/articles/czrj18yp489o)\\\\n\\\\n[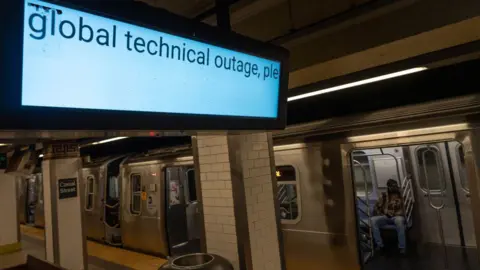\\\\\\\\\\\\n\\\\\\\\\\\\n\\'Significant number\\' of devices fixed - CrowdStrike\\\\\\\\\\\\n---------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nCybersecurity firm behind global outage says it continues to focus on restoring all impacted computers.\\\\\\\\\\\\n\\\\\\\\\\\\n13 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nBusiness](/news/articles/cgl7e33n1d0o)\\\\n\\\\n[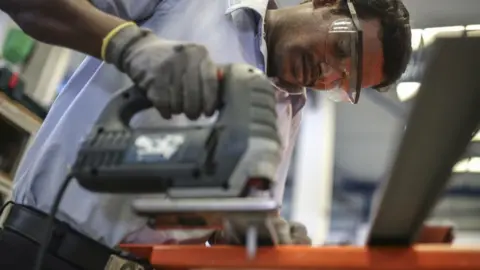\\\\\\\\\\\\n\\\\\\\\\\\\nModi\\'s new budget faces jobs crisis test in India\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nMr Modi\\'s biggest challenge in his third term will be bridging the rich-poor divide.\\\\\\\\\\\\n\\\\\\\\\\\\n16 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nAsia](/news/articles/cq5jwyel12qo)\\\\n\\\\n[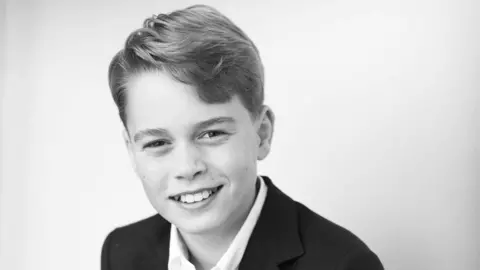\\\\\\\\\\\\n\\\\\\\\\\\\nNew Prince George photo released on 11th birthday\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nKensington Palace has posted the image, taken by his mother, Catherine, Princess of Wales.\\\\\\\\\\\\n\\\\\\\\\\\\n7 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUK](/news/articles/cw4yxd3dw7qo)\\\\n\\\\n* * *\\\\n\\\\nMust watch\\\\n----------\\\\n\\\\n[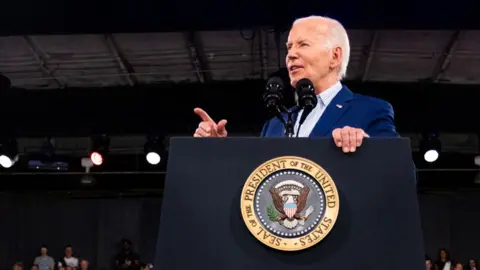\\\\\\\\\\\\n\\\\\\\\\\\\nBiden\\\\u2019s disastrous few weeks... in 90 seconds\\\\\\\\\\\\n---------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nPresident Biden has faced intense pressure to step aside since his faltering debate performance.\\\\\\\\\\\\n\\\\\\\\\\\\n17 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/videos/cx028eq4qg1o)\\\\n\\\\n[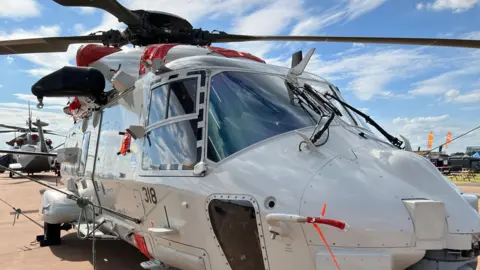\\\\\\\\\\\\n\\\\\\\\\\\\nInside the Netherlands Navy\\'s anti-submarine helicopter\\\\\\\\\\\\n-------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThe NH90 was on display at the 2024 Royal International Air Tattoo show at RAF Fairford.\\\\\\\\\\\\n\\\\\\\\\\\\n4 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nEngland](/news/videos/cmj24x03r8po)\\\\n\\\\n[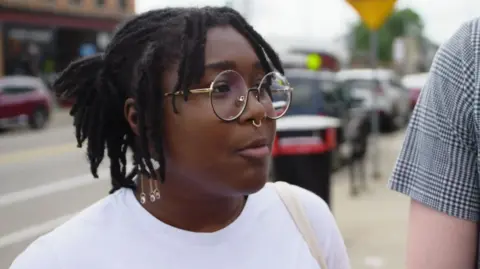\\\\\\\\\\\\n\\\\\\\\\\\\nDemocrats in Michigan react to Biden dropping out\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nDemocratic voters in Michigan give their take on Joe Biden withdrawing from the US presidential race.\\\\\\\\\\\\n\\\\\\\\\\\\n18 hrs ago](/news/videos/c51yrr2z74no)\\\\n\\\\n[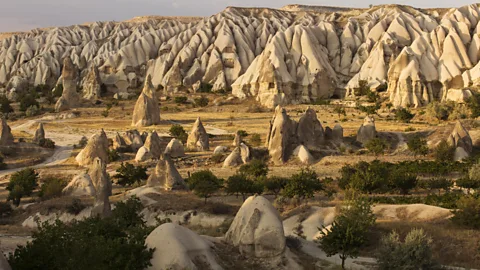\\\\\\\\\\\\n\\\\\\\\\\\\nTurkey\\'s answer to \\'Burning Man\\'\\\\\\\\\\\\n--------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nA music and art extravaganza takes place in an \\'otherworldly\\' landscape amid unique volcanic rock formations.\\\\\\\\\\\\n\\\\\\\\\\\\n13 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nCulture & Experiences](/reel/video/p0jch19q/turkey-s-answer-to-burning-man-)\\\\n\\\\n[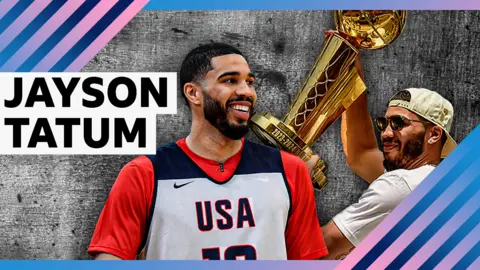\\\\\\\\\\\\n\\\\\\\\\\\\nTatum on handling criticism and \\'joy\\' of Olympics\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nTeam USA and Boston Celtics power forward Jayson Tatum says \\\\\"basketball can\\'t be your sole purpose\\\\\" as he speaks about facing criticism, and the \\\\\"joy\\\\\" that playing in the Olympics brings.\\\\\\\\\\\\n\\\\\\\\\\\\n2 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nOlympic Games](/sport/basketball/videos/cg640yk3n3zo)\\\\n\\\\n[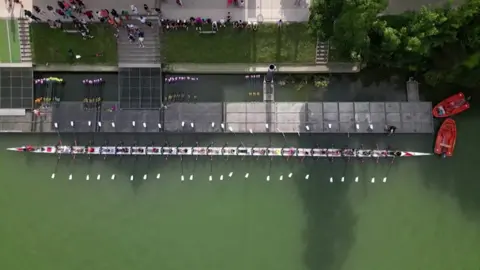\\\\\\\\\\\\n\\\\\\\\\\\\nWorld\\'s longest rowing boat to carry Olympic torch\\\\\\\\\\\\n--------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThe 24-seater boat will take the Olympic torch down a section of the River Marne on Sunday.\\\\\\\\\\\\n\\\\\\\\\\\\n1 day ago\\\\\\\\\\\\n\\\\\\\\\\\\nEurope](/news/videos/cqe6q917y1jo)\\\\n\\\\n[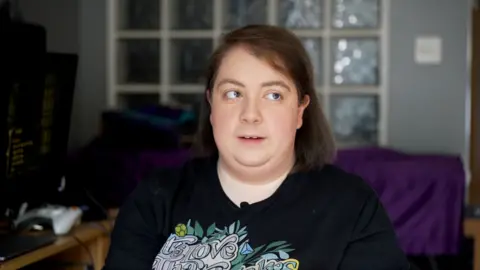\\\\\\\\\\\\n\\\\\\\\\\\\nAccessibility brings disabled gamers a sense of community\\\\\\\\\\\\n---------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nGreater accessibility in game development is opening the genre to more people with disabilities.\\\\\\\\\\\\n\\\\\\\\\\\\n1 day ago\\\\\\\\\\\\n\\\\\\\\\\\\nScotland](/news/videos/c0jq593xqk8o)\\\\n\\\\n[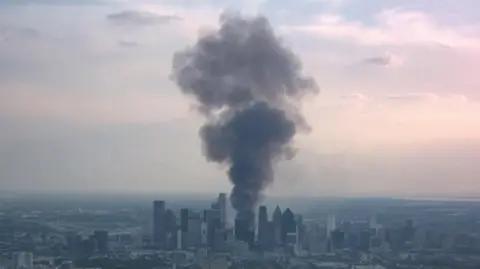\\\\\\\\\\\\n\\\\\\\\\\\\nWatch: Spire collapses as fire engulfs Texas church\\\\\\\\\\\\n---------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nA blaze at a historic church in Dallas has caused huge plumes of smoke to rise over the Texan city\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/videos/cjk325014g7o)\\\\n\\\\n* * *\\\\n\\\\nIn History\\\\n----------\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\n\\'He was after my life\\': WW1 soldier\\'s confession\\\\\\\\\\\\n------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nWorld War One broke out on 28 July, 1914. Fifty years later, one of the German soldiers, Stefan Westmann, told the BBC about his experiences fighting in the conflict.\\\\\\\\\\\\n\\\\\\\\\\\\nSee more](/culture/article/20240718-a-ww1-soldier-on-the-brutality-of-conflict)\\\\n\\\\n* * *\\\\n\\\\n[Olympic Games\\\\\\\\\\\\n-------------](https://www.bbc.com/sport/olympics)\\\\n\\\\u00a0\\\\n\\\\n[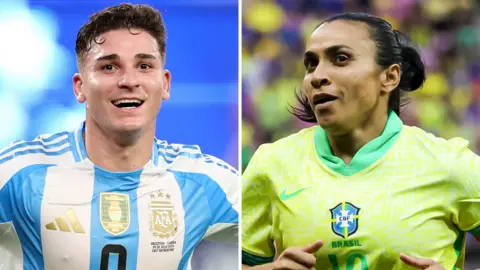\\\\\\\\\\\\n\\\\\\\\\\\\nTen footballers to watch out for at Paris Olympics\\\\\\\\\\\\n--------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nFrom Manchester City\\'s Julian Alvarez to Brazil icon Marta, BBC Sport picks out 10 footballers to watch at the Olympics.\\\\\\\\\\\\n\\\\\\\\\\\\nSee more](/sport/football/articles/cek91m98g48o)\\\\n\\\\n* * *\\\\n\\\\nUS and Canada news\\\\n------------------\\\\n\\\\n[\\'The right move but is it too late?\\' Democratic voters react\\\\\\\\\\\\n------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nJust now\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/crgk8rgm87lo)\\\\n\\\\n[Who could be Kamala Harris\\'s running mate?\\\\\\\\\\\\n------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n10 mins ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/c80ekdwk9zro)\\\\n\\\\n[Biden has backed Harris. What happens next in US election?\\\\\\\\\\\\n----------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n18 mins ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/cq5xdq71drro)\\\\n\\\\n[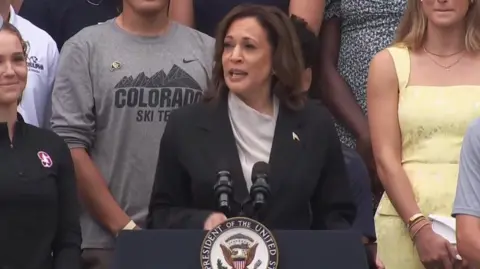\\\\\\\\\\\\n\\\\\\\\\\\\nBiden\\'s legacy of accomplishment is unmatched - Harris\\\\\\\\\\\\n------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThe US Vice-President praised Joe Biden\\'s track record as US president.\\\\\\\\\\\\n\\\\\\\\\\\\n34 mins ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/videos/c51y936y9g2o)\\\\n\\\\n* * *\\\\n\\\\nGlobal news\\\\n-----------\\\\n\\\\n[At least six killed in Croatia nursing home shooting\\\\\\\\\\\\n----------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n7 mins ago\\\\\\\\\\\\n\\\\\\\\\\\\nEurope](/news/articles/cn08d7vyj6wo)\\\\n\\\\n[Israel orders evacuation of part of Gaza humanitarian zone\\\\\\\\\\\\n----------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nMiddle East](/news/articles/cgerz8we1vgo)\\\\n\\\\n[Russian-US journalist jailed for \\'false information\\'\\\\\\\\\\\\n----------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nEurope](/news/articles/cn08d7j1qj5o)\\\\n\\\\n[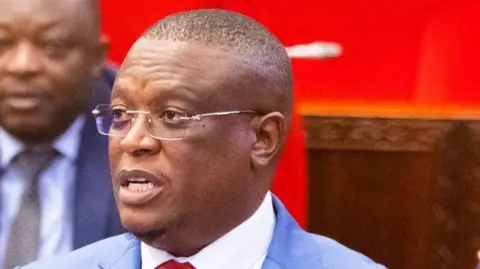\\\\\\\\\\\\n\\\\\\\\\\\\nTanzanian minister sacked after poll rigging remarks\\\\\\\\\\\\n----------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nNape Nnauye caused outrage for saying he could help an MP rig elections - comments, he said, he made in jest.\\\\\\\\\\\\n\\\\\\\\\\\\n3 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nAfrica](/news/articles/cd1e48677w0o)\\\\n\\\\n* * *\\\\n\\\\nUK news\\\\n-------\\\\n\\\\n[Campaigners in court over Magna Carta incident\\\\\\\\\\\\n----------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n54 mins ago\\\\\\\\\\\\n\\\\\\\\\\\\nUK](/news/articles/cxw29lg4y8go)\\\\n\\\\n[New Prince George photo released on 11th birthday\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n7 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUK](/news/articles/cw4yxd3dw7qo)\\\\n\\\\n[\\'We have one of the best comedy scenes in the UK\\'\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n12 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUK](/news/articles/c4ngrdpwlx3o)\\\\n\\\\n[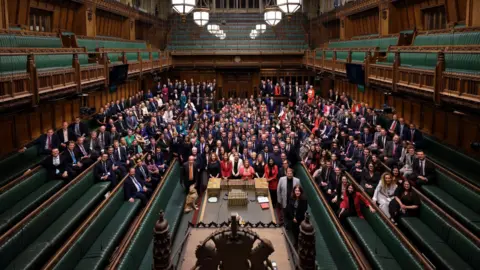\\\\\\\\\\\\n\\\\\\\\\\\\nHow does a surprise MP prepare for life in office?\\\\\\\\\\\\n--------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nNew MPs in the East describe the experience of unexpectedly picking up the reins of public life.\\\\\\\\\\\\n\\\\\\\\\\\\n12 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nEngland](/news/articles/cxr2g7rk3dxo)\\\\n\\\\n* * *\\\\n\\\\nSport\\\\n-----\\\\n\\\\n[Ferdinand\\'s persuasive powers sealed Man Utd\\'s Yoro deal\\\\\\\\\\\\n--------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n49 mins ago\\\\\\\\\\\\n\\\\\\\\\\\\nMan Utd](/sport/football/articles/c9e950ev0xpo)\\\\n\\\\n[Aston Villa complete \\\\u00a350m deal for Everton\\'s Onana\\\\\\\\\\\\n--------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nPremier League](/sport/football/articles/cgrlgzx6gpro)\\\\n\\\\n[Australia would not pick convicted rapist Olympian\\\\\\\\\\\\n--------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n3 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nOlympic Games](/sport/articles/c2v0j0j6nqlo)\\\\n\\\\n[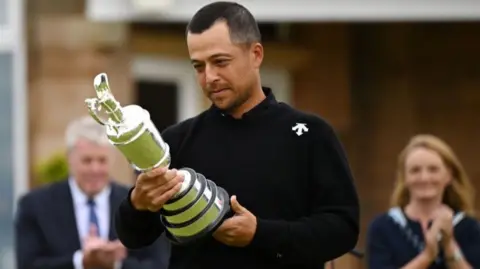\\\\\\\\\\\\n\\\\\\\\\\\\n\\'Schauffele passes ultimate examination in classic Open\\'\\\\\\\\\\\\n--------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThe 152nd Open should be remembered as a classic, writes BBC golf correspondent Iain Carter.\\\\\\\\\\\\n\\\\\\\\\\\\n1 hr ago\\\\\\\\\\\\n\\\\\\\\\\\\nGolf](/sport/golf/articles/cv2g1glwypqo)\\\\n\\\\n* * *\\\\n\\\\n[Video\\\\\\\\\\\\n-----](https://www.bbc.com/video)\\\\n\\\\u00a0\\\\n\\\\n[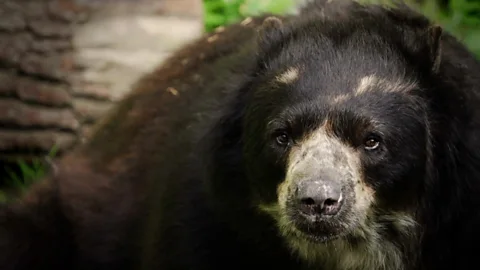\\\\\\\\\\\\n\\\\\\\\\\\\nThe \\'Paddington bears\\' facing climate threat\\\\\\\\\\\\n--------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nDrought forces the real Paddington Bear\\\\u00a0into deadly conflict with cattle farmers in the Andes.\\\\\\\\\\\\n\\\\\\\\\\\\nSee more](/reel/video/p0jbv222/climate-chaos-makes-paddington-bear-hangry-)\\\\n\\\\n* * *\\\\n\\\\nBusiness\\\\n--------\\\\n\\\\n[Ryanair set to slash summer fares as profits drop\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n8 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nBusiness](/news/articles/cj50d6q3jlro)\\\\n\\\\n[Former chancellor Zahawi mulling bid for the Telegraph\\\\\\\\\\\\n------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n26 mins ago\\\\\\\\\\\\n\\\\\\\\\\\\nBusiness](/news/articles/c4ng5q4jd62o)\\\\n\\\\n[Prime sued in trademark case by US Olympic committee\\\\\\\\\\\\n----------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n1 day ago](/news/articles/c4ng785gjv0o)\\\\n\\\\n[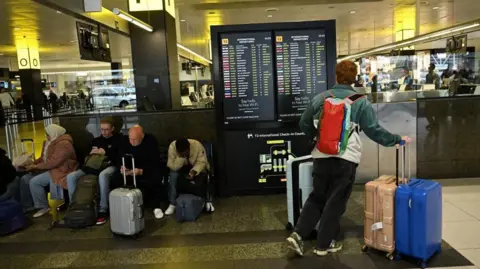\\\\\\\\\\\\n\\\\\\\\\\\\nCrowdStrike IT outage affected 8.5 million Windows devices, Microsoft says\\\\\\\\\\\\n--------------------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nIt\\\\u2019s the first time that a number has been put on the glitch that is still causing problems around the world.\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nTechnology](/news/articles/cpe3zgznwjno)\\\\n\\\\n* * *\\\\n\\\\nInnovation\\\\n----------\\\\n\\\\n[Company wins funding to make medicine in space\\\\\\\\\\\\n----------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n4 hrs ago](/news/articles/cp9vdxwjddeo)\\\\n\\\\n[Summer surge: why Covid-19 isn\\'t yet seasonal\\\\\\\\\\\\n---------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n1 day ago\\\\\\\\\\\\n\\\\\\\\\\\\nFuture](/future/article/20240719-why-covid-19-is-spreading-this-summer)\\\\n\\\\n[Scam warning as fake emails and websites target users after outage\\\\\\\\\\\\n------------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nTechnology](/news/articles/cq5xy12pynyo)\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\nAre fermented foods actually good for our health?\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nWhile humans have been eating fermented foods since ancient times, researchers are only starting to unravel some of the biggest questions about their health benefits.\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nFuture](/future/article/20240719-are-fermented-foods-actually-good-for-you)\\\\n\\\\n* * *\\\\n\\\\nCulture\\\\n-------\\\\n\\\\n[How to choose the most eco-friendly swimwear\\\\\\\\\\\\n--------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nCulture](/culture/article/20240719-how-to-choose-the-best-and-most-eco-friendly-swimwear)\\\\n\\\\n[In pictures: Colonial India through the eyes of foreign artists\\\\\\\\\\\\n---------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nAsia](/news/articles/c28ejl4nvgyo)\\\\n\\\\n[Auction for John Lennon glasses and Abbey Road photos\\\\\\\\\\\\n-----------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n1 day ago\\\\\\\\\\\\n\\\\\\\\\\\\nSurrey](/news/articles/cp085ym9l3ro)\\\\n\\\\n[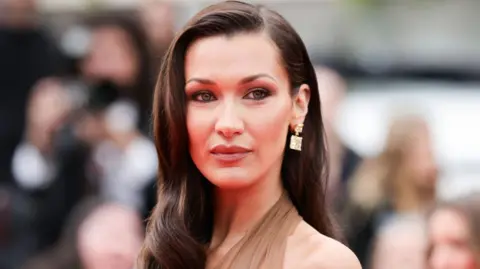\\\\\\\\\\\\n\\\\\\\\\\\\nBella Hadid\\'s Adidas advert dropped after Israeli criticism\\\\\\\\\\\\n-----------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThe model was chosen to promote shoes referencing the 1972 Munich Olympics, at which 11 Israeli athletes were killed.\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nCulture](/news/articles/ceqdwpv8vw1o)\\\\n\\\\n* * *\\\\n\\\\nTravel\\\\n------\\\\n\\\\n[The Indian dish Kamala Harris loves\\\\\\\\\\\\n-----------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n1 hr ago\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20201026-dosa-indias-wholesome-fast-food-obsession)\\\\n\\\\n[Italy\\'s most iconic trail reopens after 12 years\\\\\\\\\\\\n------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n3 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240722-cinque-terre-italys-path-of-love-reopens-after-12-years)\\\\n\\\\n[The US\\'s little-known \\'Ellis Island of the West\\'\\\\\\\\\\\\n------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n5 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240719-angel-island-the-little-known-ellis-island-of-the-west-us)\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\nThe Turkish ice cream eaten with a knife and fork\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nDondurma isn\\'t like any other ice cream you\\'ll find, and the epicentre of its production is still reeling from the powerful earthquakes that decimated the nation.\\\\\\\\\\\\n\\\\\\\\\\\\n1 day ago\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240719-dondurma-the-turkish-ice-cream-eaten-with-a-knife-and-fork)\\\\n\\\\n* * *\\\\n\\\\n[Travel\\\\\\\\\\\\n------](https://www.bbc.com/travel)\\\\n\\\\u00a0\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\nThe US\\'s little-known \\'Ellis Island of the West\\'\\\\\\\\\\\\n------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThis former quarantine and military station once processed as many as one million immigrants. Now, the picturesque island is one of the San Francisco Bay Area\\'s best urban getaways.\\\\\\\\\\\\n\\\\\\\\\\\\nSee more](/travel/article/20240719-angel-island-the-little-known-ellis-island-of-the-west-us)\\\\n\\\\n* * *\\\\n\\\\nSign up for our newsletters\\\\n---------------------------\\\\n\\\\n[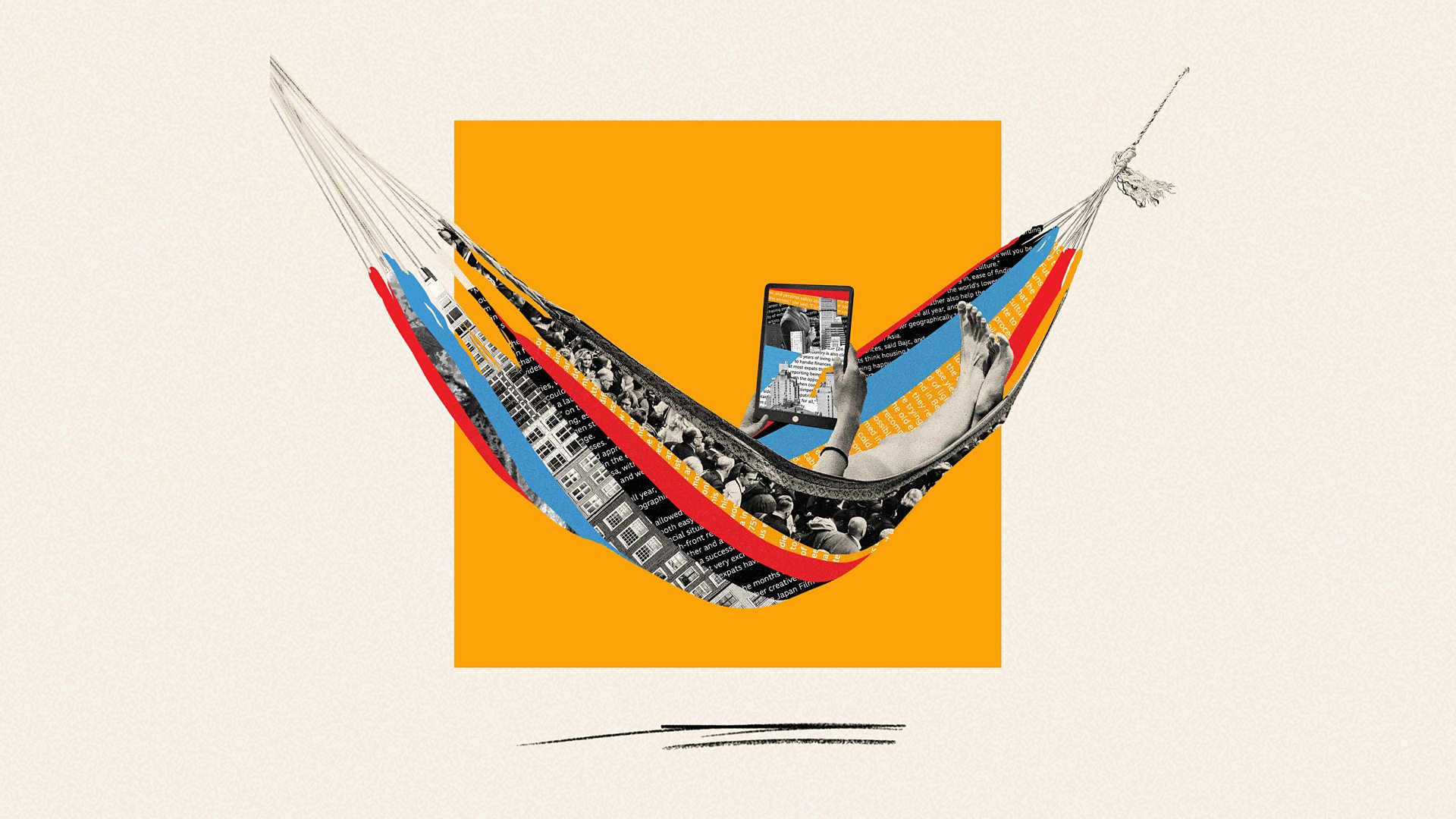\\\\\\\\\\\\n\\\\\\\\\\\\nThe Essential List\\\\\\\\\\\\n------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThe week\\'s best stories, handpicked by BBC editors, in your inbox every Tuesday and Friday.](https://cloud.email.bbc.com/SignUp10_08?&at_bbc_team=studios&at_medium=display&at_objective=acquisition&at_ptr_type=&at_ptr_name=bbc.comhp&at_format=Module&at_link_origin=homepage&at_campaign=essentiallist&at_campaign_type=owned)\\\\n\\\\n[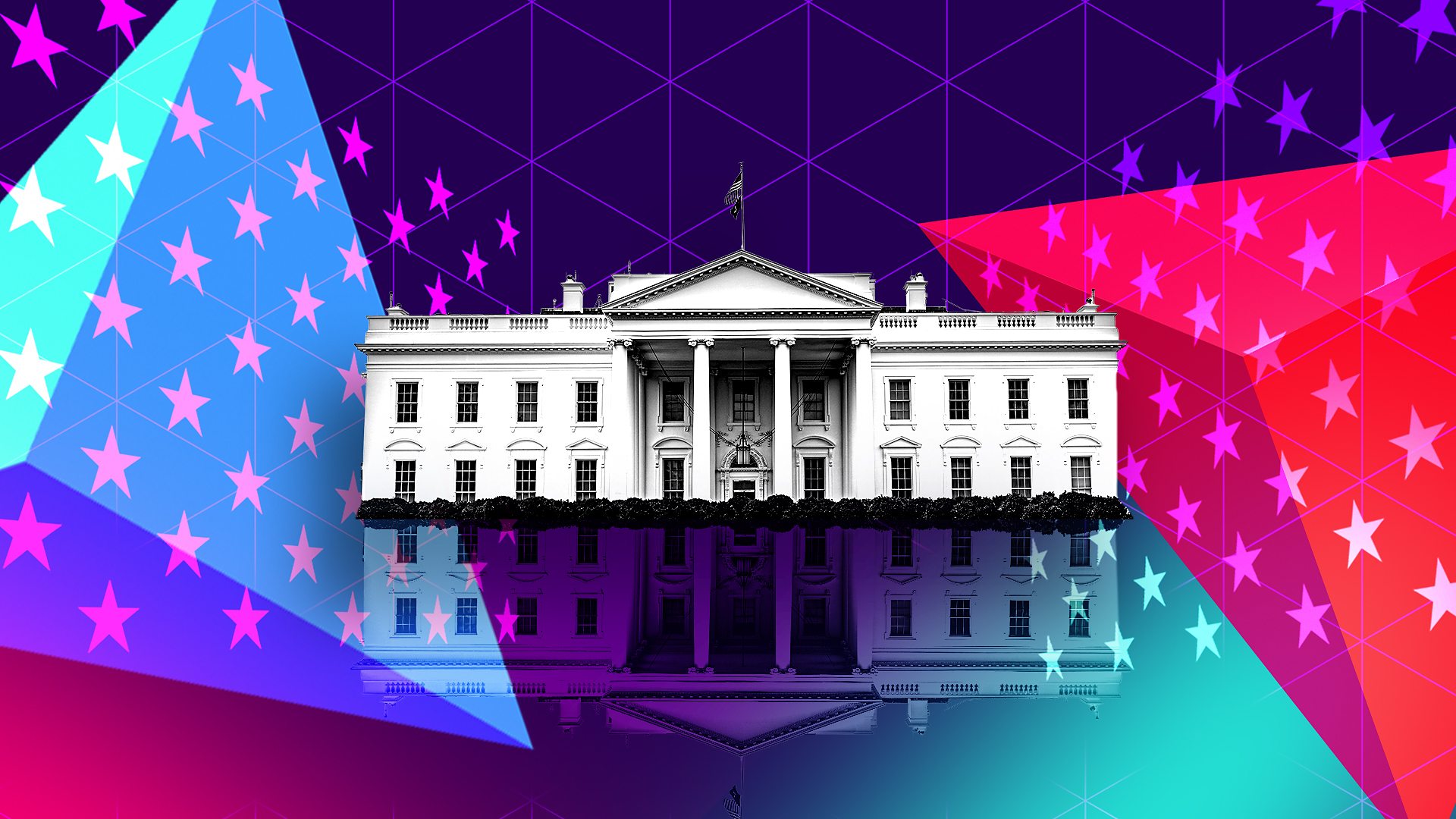\\\\\\\\\\\\n\\\\\\\\\\\\nUS Election Unspun\\\\\\\\\\\\n------------------\\\\\\\\\\\\n\\\\\\\\\\\\nCut through the spin with North America correspondent Anthony Zurcher - in your inbox every Wednesday.](https://cloud.email.bbc.com/US_Election_Unspun_newsletter_signup?&at_bbc_team=studios&at_medium=display&at_objective=acquisition&at_ptr_type=&at_ptr_name=bbc.comhp&at_format=Module&at_link_origin=homepage&at_campaign=uselectionunspun&at_campaign_type=owned)\\\\n\\\\n[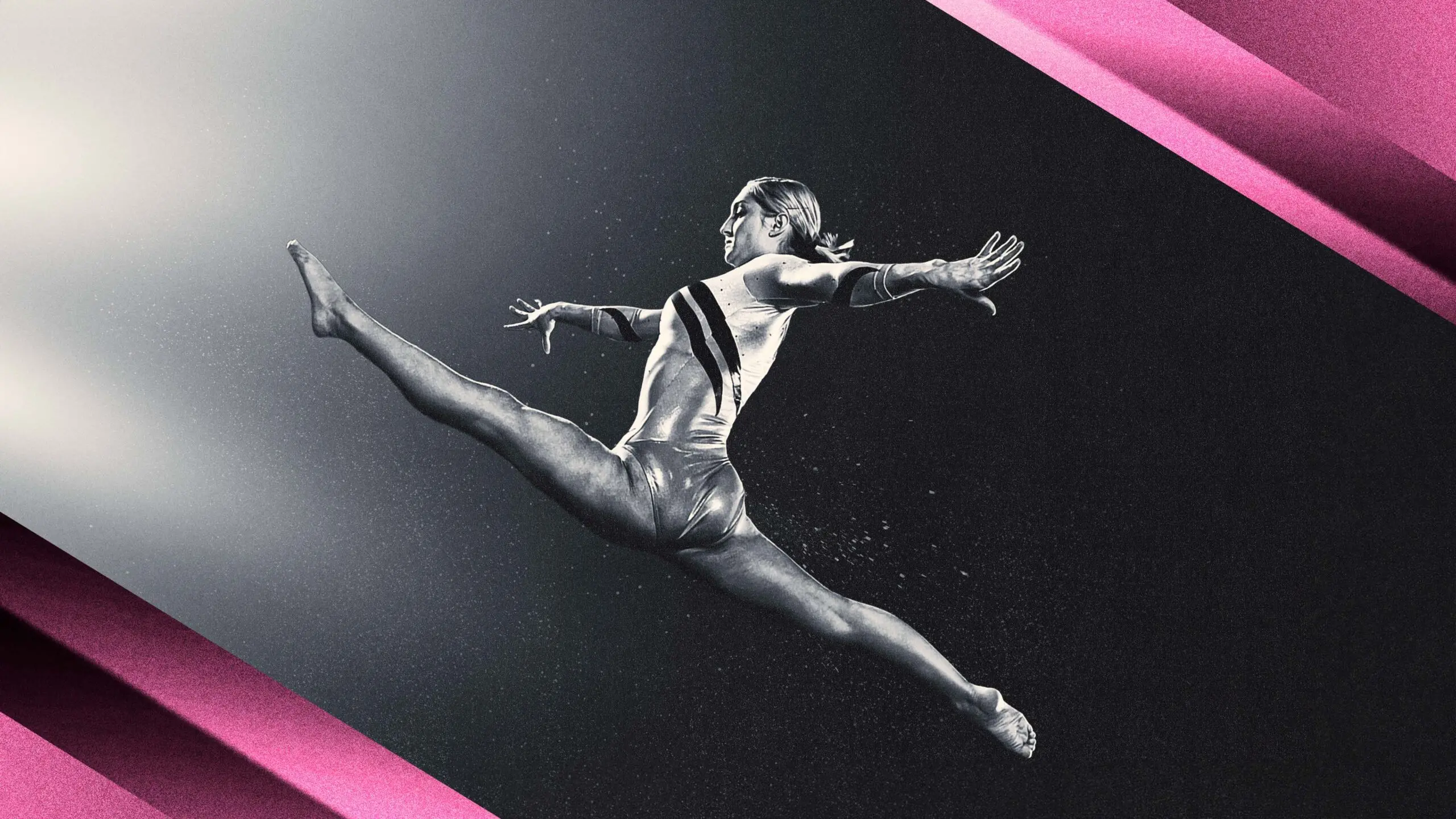\\\\\\\\\\\\n\\\\\\\\\\\\nMedal Moments\\\\\\\\\\\\n-------------\\\\\\\\\\\\n\\\\\\\\\\\\nYour global guide to the Paris Olympics, from key highlights to heroic stories, daily to your inbox throughout the Games.](https://cloud.email.bbc.com/medalmoments_newsletter_signup?&at_bbc_team=studios&at_medium=emails&at_objective=acquisition&at_ptr_type=&at_ptr_name=bbc.com&at_format=Module&at_link_origin=homepage&at_campaign=olympics2024&at_campaign_id=&at_adset_name=&at_adset_id=&at_creation=&at_creative_id=&at_campaign_type=owned)\\\\n\\\\n* * *\\\\n\\\\nEarth\\\\n-----\\\\n\\\\n[The \\'Paddington bears\\' facing climate threat\\\\\\\\\\\\n--------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nNatural wonders](/reel/video/p0jbv222/climate-chaos-makes-paddington-bear-hangry-)\\\\n\\\\n[The simple Japanese method for a tidier fridge\\\\\\\\\\\\n----------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n3 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nFuture](/future/article/20240715-the-simple-japanese-method-for-a-tidier-and-less-wasteful-fridge)\\\\n\\\\n[Conspiracy theories swirl about geo-engineering, but could it help save the planet?\\\\\\\\\\\\n-----------------------------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nBBC InDepth](/news/articles/c98qp79gj4no)\\\\n\\\\n[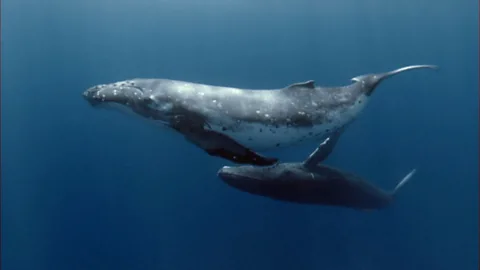\\\\\\\\\\\\n\\\\\\\\\\\\nFace to face with humpback whales\\\\\\\\\\\\n---------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nReece Parkinson discovers how locals are protecting their stunning marine environment.\\\\\\\\\\\\n\\\\\\\\\\\\n3 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/reel/video/p0jbpt60/face-to-face-with-humpback-whales)\\\\n\\\\n* * *\\\\n\\\\nScience and health\\\\n------------------\\\\n\\\\n[India alert after boy dies from Nipah virus in Kerala\\\\\\\\\\\\n-----------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n6 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nAsia](/news/articles/cj50d7e9vp6o)\\\\n\\\\n[Are fermented foods actually good for our health?\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nFuture](/future/article/20240719-are-fermented-foods-actually-good-for-you)\\\\n\\\\n[Summer surge: why Covid-19 isn\\'t yet seasonal\\\\\\\\\\\\n---------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n1 day ago\\\\\\\\\\\\n\\\\\\\\\\\\nFuture](/future/article/20240719-why-covid-19-is-spreading-this-summer)\\\\n\\\\n[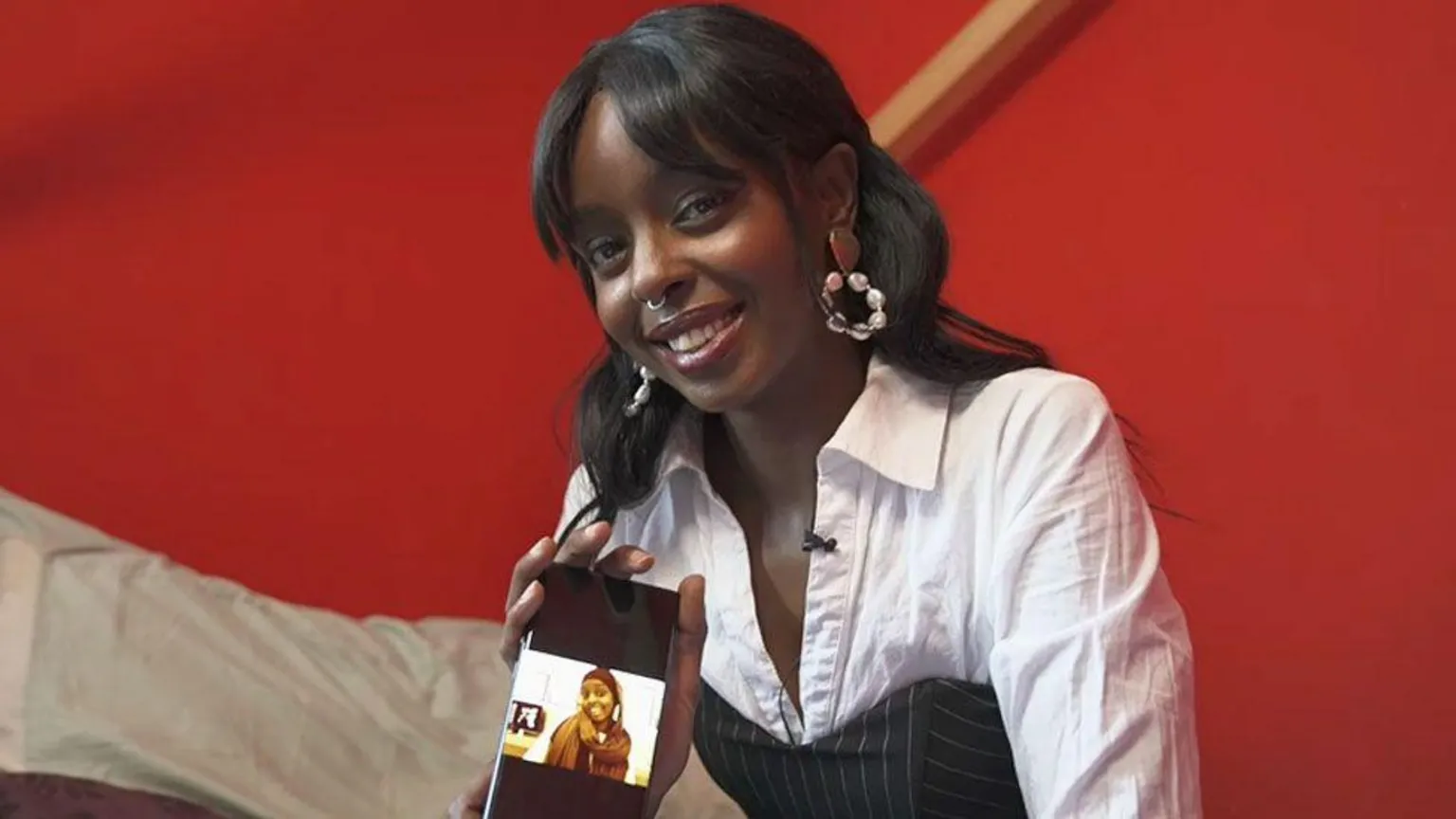\\\\\\\\\\\\n\\\\\\\\\\\\n\\'A way to fight back\\': FGM survivors reclaim vulvas with surgery\\\\\\\\\\\\n----------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nIn Somalia, it\\'s believed cutting off a girl\\'s outer genitalia will guarantee their virginity.\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nAfrica](/news/articles/cyx0perl8yno)\\\\n\\\\n* * *\\\\n\\\\nWorld\\\\u2019s Table\\\\n-------------\\\\n\\\\n[The Turkish ice cream eaten with a knife and fork\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n1 day ago\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240719-dondurma-the-turkish-ice-cream-eaten-with-a-knife-and-fork)\\\\n\\\\n[India\\'s cooling drinks to beat the heat\\\\\\\\\\\\n---------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n7 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240715-gond-katira-a-natural-way-to-cool-down-in-indias-scorching-summers)\\\\n\\\\n[The world\\'s biggest restaurant is coming to Paris\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n9 Jul 2024\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240708-paris-olympics-2024-worlds-biggest-restaurant)\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\nThe wild ceremonies of the Turkish \\'meatball\\'\\\\\\\\\\\\n---------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nOne of the country\\'s most popular fast-food items, \\\\u00e7i\\\\u011f k\\\\u00f6fte is traditionally associated with wild and rowdy gatherings in south-eastern Turkey.\\\\\\\\\\\\n\\\\\\\\\\\\n1 Jun 2024\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240531-the-wild-ceremonies-surrounding-a-turkish-meatball)\\\\n\\\\n* * *\\\\n\\\\nThe Specialist\\\\n--------------\\\\n\\\\n[Guide to Helsinki\\'s happiest places\\\\\\\\\\\\n-----------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240718-a-happiness-hackers-guide-to-the-happiest-outdoor-places-in-helsinki)\\\\n\\\\n[A pastry chef\\'s favourite bakeries in Paris\\\\\\\\\\\\n-------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n5 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240717-david-lebovitzs-ultimate-guide-to-the-best-bakeries-in-paris-right-now)\\\\n\\\\n[Chef Andrew Zimmern\\'s favourite US restaurants\\\\\\\\\\\\n----------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n14 Jul 2024\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240714-chef-andrew-zimmerns-favourite-us-restaurants)\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\nA First Lady\\'s guide to Iceland\\\\\\\\\\\\n-------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nEliza Reid moved to Iceland 20 years ago for love and now she\\'s the First Lady. Here are her favourite ways to enjoy a \\\\\"chill\\\\\" Icelandic weekend.\\\\\\\\\\\\n\\\\\\\\\\\\n11 Jul 2024\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240710-icelands-first-lady-takes-you-on-a-tour-of-her-super-chill-nation)\\\\n\\\\n* * *\\\\n\\\\n[British Broadcasting Corporation](/)\\\\n\\\\n* [Home](https://www.bbc.com/)\\\\n \\\\n* [News](/news)\\\\n \\\\n* [Sport](/sport)\\\\n \\\\n* [Business](/business)\\\\n \\\\n* [Innovation](/innovation)\\\\n \\\\n* [Culture](/culture)\\\\n \\\\n* [Travel](/travel)\\\\n \\\\n* [Earth](/future-planet)\\\\n \\\\n* [Video](/video)\\\\n \\\\n* [Live](/live)\\\\n \\\\n* [Audio](https://www.bbc.co.uk/sounds)\\\\n \\\\n* [Weather](https://www.bbc.com/weather)\\\\n \\\\n* [BBC Shop](https://shop.bbc.com/)\\\\n \\\\n\\\\nBBC in other languages\\\\n\\\\nFollow BBC on:\\\\n--------------\\\\n\\\\n* [Terms of Use](https://www.bbc.co.uk/usingthebbc/terms)\\\\n \\\\n* [About the BBC](https://www.bbc.co.uk/aboutthebbc)\\\\n \\\\n* [Privacy Policy](https://www.bbc.com/usingthebbc/privacy/)\\\\n \\\\n* [Cookies](https://www.bbc.com/usingthebbc/cookies/)\\\\n \\\\n* [Accessibility Help](https://www.bbc.co.uk/accessibility/)\\\\n \\\\n* [Contact the BBC](https://www.bbc.co.uk/contact)\\\\n \\\\n* [Advertise with us](https://www.bbc.com/advertisingcontact)\\\\n \\\\n* [Do not share or sell my info](https://www.bbc.com/usingthebbc/cookies/how-can-i-change-my-bbc-cookie-settings/)\\\\n \\\\n* [Contact technical support](https://www.bbc.com/contact-bbc-com-help)\\\\n \\\\n\\\\nCopyright 2024 BBC. All rights reserved.\\\\u00a0\\\\u00a0The _BBC_ is _not responsible for the content of external sites._\\\\u00a0[**Read about our approach to external linking.**](https://www.bbc.co.uk/editorialguidelines/guidance/feeds-and-links)\", \"markdown\": \"\\\\n\\\\n[British Broadcasting Corporation](/)\\\\n\\\\n[Watch Live](/watch-live-news)\\\\n\\\\nRegisterSign In\\\\n\\\\n* [Home](/)\\\\n \\\\n* [News](/news)\\\\n \\\\n* [Sport](/sport)\\\\n \\\\n* [Business](/business)\\\\n \\\\n* [Innovation](/innovation)\\\\n \\\\n* [Culture](/culture)\\\\n \\\\n* [Travel](/travel)\\\\n \\\\n* [Earth](/future-planet)\\\\n \\\\n* [Video](/video)\\\\n \\\\n* [Live](/live)\\\\n \\\\n\\\\nRegisterSign In\\\\n\\\\n[Home](/)\\\\n\\\\nNews\\\\n\\\\n[Sport](/sport)\\\\n\\\\nBusiness\\\\n\\\\nInnovation\\\\n\\\\nCulture\\\\n\\\\nTravel\\\\n\\\\nEarth\\\\n\\\\n[Video](/video)\\\\n\\\\nLive\\\\n\\\\n[Audio](https://www.bbc.co.uk/sounds)\\\\n\\\\n[Weather](https://www.bbc.com/weather)\\\\n\\\\n[Newsletters](https://www.bbc.com/newsletters)\\\\n\\\\n[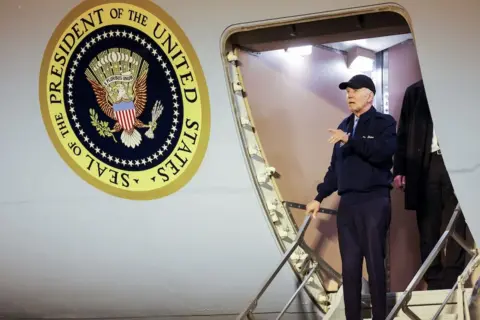\\\\\\\\\\\\n\\\\\\\\\\\\nThe last days of the Biden campaign \\\\u2013 BBC correspondent\\\\u2019s account\\\\\\\\\\\\n-----------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nBBC correspondent Tom Bateman takes us behind the scenes in the final days of Biden\\'s campaign.\\\\\\\\\\\\n\\\\\\\\\\\\n3 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/c250zqgrpqgo)\\\\n\\\\n[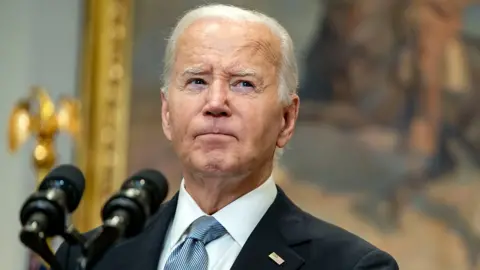\\\\\\\\\\\\n\\\\\\\\\\\\nIsolating at a beach house, Biden gave aides one minute notice of exit\\\\\\\\\\\\n----------------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nMost of the president\\'s senior aides found out about his decision to exit the race minutes before he publicly announced it.\\\\\\\\\\\\n\\\\\\\\\\\\n15 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/c3gdzmdje5xo)\\\\n\\\\n[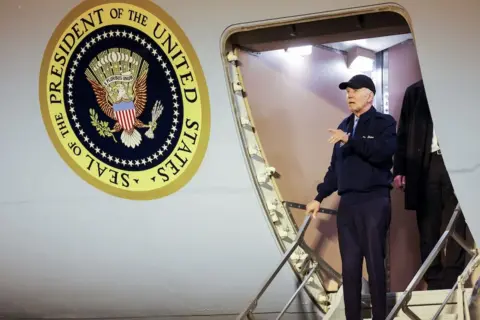\\\\\\\\\\\\n\\\\\\\\\\\\nThe last days of the Biden campaign \\\\u2013 BBC correspondent\\\\u2019s account\\\\\\\\\\\\n-----------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nBBC correspondent Tom Bateman takes us behind the scenes in the final days of Biden\\'s campaign.\\\\\\\\\\\\n\\\\\\\\\\\\n3 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/c250zqgrpqgo)\\\\n\\\\n[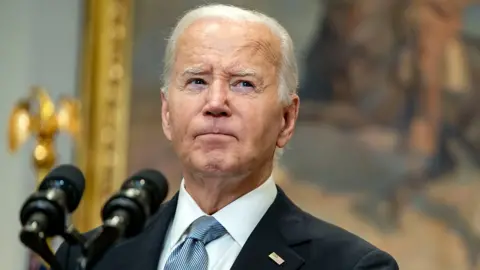\\\\\\\\\\\\n\\\\\\\\\\\\nIsolating at a beach house, Biden gave aides one minute notice of exit\\\\\\\\\\\\n----------------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nMost of the president\\'s senior aides found out about his decision to exit the race minutes before he publicly announced it.\\\\\\\\\\\\n\\\\\\\\\\\\n15 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/c3gdzmdje5xo)\\\\n\\\\n[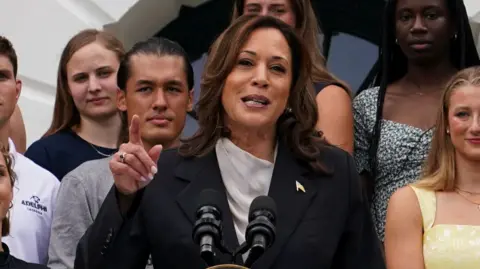\\\\\\\\\\\\n\\\\\\\\\\\\nLIVE\\\\\\\\\\\\n\\\\\\\\\\\\nKamala Harris speaks for first time since Biden left race - as endorsements mount\\\\\\\\\\\\n---------------------------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThe US vice-president appears at the White House for a pre-scheduled event - as key Democrats line up to back her candidacy.](https://www.bbc.com/news/live/cv2gryx1yx1t)\\\\n\\\\n* * *\\\\n\\\\n[What Biden quitting means for Harris, the Democrats and Trump\\\\\\\\\\\\n-------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nPresident Biden has upended the 2024 White House race for the Democrats. Here is what it means for Kamala Harris, his party and Trump.\\\\\\\\\\\\n\\\\\\\\\\\\n19 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/cpwd8yzw45qo)\\\\n\\\\n[Who could be Kamala Harris\\'s running mate?\\\\\\\\\\\\n------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nIt\\\\u2019s not a done deal but some potential rivals have quickly thrown their support behind her.\\\\\\\\\\\\n\\\\\\\\\\\\n10 mins ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/c80ekdwk9zro)\\\\n\\\\n[LIVE\\\\\\\\\\\\n\\\\\\\\\\\\nTensions flare as Congress presses Secret Service boss on \\'failed\\' Trump rally security\\\\\\\\\\\\n---------------------------------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nMembers of both parties have called for Kimberly Cheatle to resign in a House committee hearing that is seeking answers over security failures at the rally on 13 July.](https://www.bbc.com/news/live/c724wqpy4qnt)\\\\n\\\\n[The president\\'s protectors are hardly noticeable - until things go wrong\\\\\\\\\\\\n------------------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThe attempted assassination of Donald Trump has raised questions about the Secret Service\\'s record.\\\\\\\\\\\\n\\\\\\\\\\\\n6 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/c16j896003xo)\\\\n\\\\n* * *\\\\n\\\\nOnly from the BBC\\\\n-----------------\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\nWhy you are probably sitting for too long\\\\\\\\\\\\n-----------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nSitting down is ingrained in most peoples\\' days. But staying sedentary for too long can increase the risk of serious health conditions like cardiovascular disease and type 2 diabetes.\\\\\\\\\\\\n\\\\\\\\\\\\n4 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nFuture](/future/article/20240722-why-you-are-probably-sitting-down-for-too-long)\\\\n\\\\n[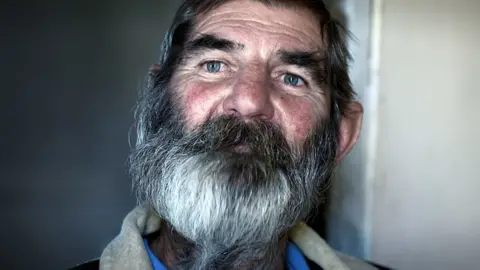\\\\\\\\\\\\n\\\\\\\\\\\\nMass killer who \\\\u2018hunted\\\\u2019 black people says police encouraged him\\\\\\\\\\\\n----------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nEx-security guard, Louis van Schoor, killed dozens in South Africa but was only jailed for seven murders.\\\\\\\\\\\\n\\\\\\\\\\\\n17 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nAfrica](/news/articles/c51yqdy3q61o)\\\\n\\\\n* * *\\\\n\\\\n[More news\\\\\\\\\\\\n---------](https://www.bbc.com/news)\\\\n\\\\u00a0\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\nUAE jails 57 Bangladeshis over protests against own government\\\\\\\\\\\\n--------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThree defendants were sentenced to life after being convicted of \\\\\"inciting riots\\\\\" in the Gulf state.\\\\\\\\\\\\n\\\\\\\\\\\\n5 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nWorld](/news/articles/crgk8gnpg0zo)\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\nIsrael orders evacuation of part of Gaza humanitarian zone\\\\\\\\\\\\n----------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nStrikes are reported near Khan Younis, after people are told to leave eastern areas in the zone.\\\\\\\\\\\\n\\\\\\\\\\\\n2 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nMiddle East](/news/articles/cgerz8we1vgo)\\\\n\\\\n[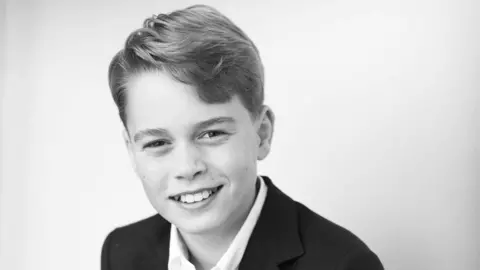\\\\\\\\\\\\n\\\\\\\\\\\\nNew Prince George photo released on 11th birthday\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nKensington Palace has posted the image, taken by his mother, Catherine, Princess of Wales.\\\\\\\\\\\\n\\\\\\\\\\\\n7 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUK](/news/articles/cw4yxd3dw7qo)\\\\n\\\\n[Piastri wins in Hungary after Norris team orders row\\\\\\\\\\\\n----------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nOscar Piastri takes his maiden grand prix victory ahead of McLaren team-mate Lando Norris in a dramatic race in Hungary.\\\\\\\\\\\\n\\\\\\\\\\\\n1 day ago\\\\\\\\\\\\n\\\\\\\\\\\\nFormula 1](/sport/formula1/articles/cg3jzy3e8q1o)\\\\n\\\\n[India alert after boy dies from Nipah virus in Kerala\\\\\\\\\\\\n-----------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThe virus is transmitted from animals such as pigs and fruit bats to humans.\\\\\\\\\\\\n\\\\\\\\\\\\n6 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nAsia](/news/articles/cj50d7e9vp6o)\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\nIsrael orders evacuation of part of Gaza humanitarian zone\\\\\\\\\\\\n----------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nStrikes are reported near Khan Younis, after people are told to leave eastern areas in the zone.\\\\\\\\\\\\n\\\\\\\\\\\\n2 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nMiddle East](/news/articles/cgerz8we1vgo)\\\\n\\\\n[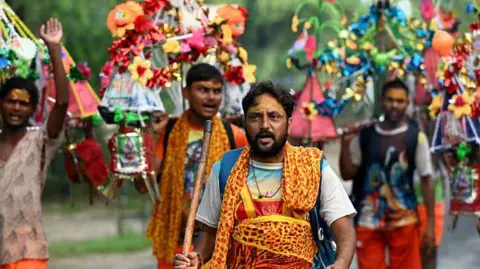\\\\\\\\\\\\n\\\\\\\\\\\\nIndia court blocks order for eateries to display owners\\' names\\\\\\\\\\\\n--------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nCritics say ordering restaurants to prominently display names of owners is discriminatory towards Muslims.\\\\\\\\\\\\n\\\\\\\\\\\\n6 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nAsia](/news/articles/czrj18yp489o)\\\\n\\\\n[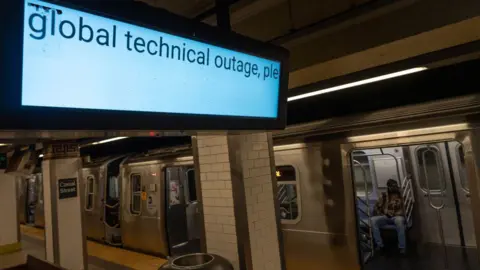\\\\\\\\\\\\n\\\\\\\\\\\\n\\'Significant number\\' of devices fixed - CrowdStrike\\\\\\\\\\\\n---------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nCybersecurity firm behind global outage says it continues to focus on restoring all impacted computers.\\\\\\\\\\\\n\\\\\\\\\\\\n13 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nBusiness](/news/articles/cgl7e33n1d0o)\\\\n\\\\n[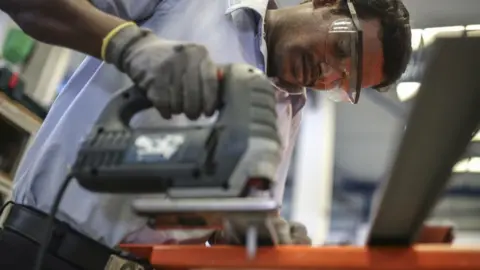\\\\\\\\\\\\n\\\\\\\\\\\\nModi\\'s new budget faces jobs crisis test in India\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nMr Modi\\'s biggest challenge in his third term will be bridging the rich-poor divide.\\\\\\\\\\\\n\\\\\\\\\\\\n16 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nAsia](/news/articles/cq5jwyel12qo)\\\\n\\\\n[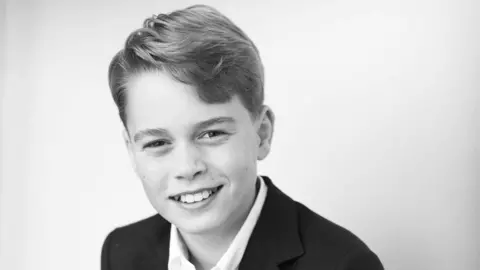\\\\\\\\\\\\n\\\\\\\\\\\\nNew Prince George photo released on 11th birthday\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nKensington Palace has posted the image, taken by his mother, Catherine, Princess of Wales.\\\\\\\\\\\\n\\\\\\\\\\\\n7 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUK](/news/articles/cw4yxd3dw7qo)\\\\n\\\\n* * *\\\\n\\\\nMust watch\\\\n----------\\\\n\\\\n[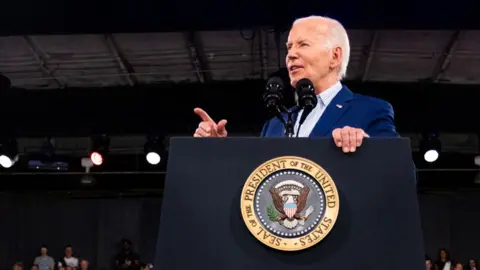\\\\\\\\\\\\n\\\\\\\\\\\\nBiden\\\\u2019s disastrous few weeks... in 90 seconds\\\\\\\\\\\\n---------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nPresident Biden has faced intense pressure to step aside since his faltering debate performance.\\\\\\\\\\\\n\\\\\\\\\\\\n17 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/videos/cx028eq4qg1o)\\\\n\\\\n[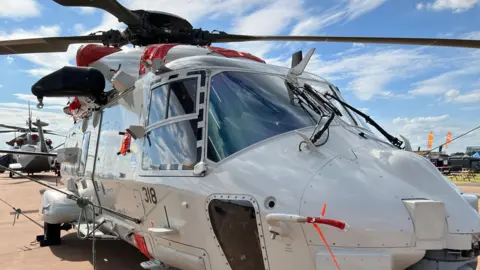\\\\\\\\\\\\n\\\\\\\\\\\\nInside the Netherlands Navy\\'s anti-submarine helicopter\\\\\\\\\\\\n-------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThe NH90 was on display at the 2024 Royal International Air Tattoo show at RAF Fairford.\\\\\\\\\\\\n\\\\\\\\\\\\n4 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nEngland](/news/videos/cmj24x03r8po)\\\\n\\\\n[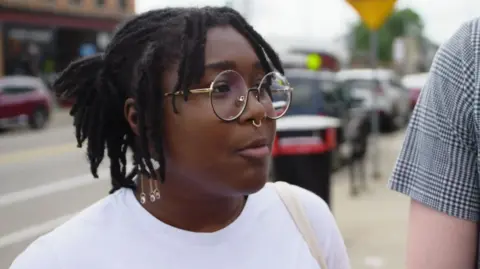\\\\\\\\\\\\n\\\\\\\\\\\\nDemocrats in Michigan react to Biden dropping out\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nDemocratic voters in Michigan give their take on Joe Biden withdrawing from the US presidential race.\\\\\\\\\\\\n\\\\\\\\\\\\n18 hrs ago](/news/videos/c51yrr2z74no)\\\\n\\\\n[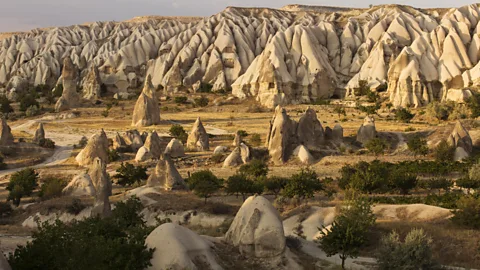\\\\\\\\\\\\n\\\\\\\\\\\\nTurkey\\'s answer to \\'Burning Man\\'\\\\\\\\\\\\n--------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nA music and art extravaganza takes place in an \\'otherworldly\\' landscape amid unique volcanic rock formations.\\\\\\\\\\\\n\\\\\\\\\\\\n13 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nCulture & Experiences](/reel/video/p0jch19q/turkey-s-answer-to-burning-man-)\\\\n\\\\n[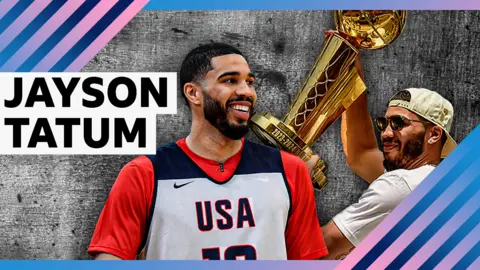\\\\\\\\\\\\n\\\\\\\\\\\\nTatum on handling criticism and \\'joy\\' of Olympics\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nTeam USA and Boston Celtics power forward Jayson Tatum says \\\\\"basketball can\\'t be your sole purpose\\\\\" as he speaks about facing criticism, and the \\\\\"joy\\\\\" that playing in the Olympics brings.\\\\\\\\\\\\n\\\\\\\\\\\\n2 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nOlympic Games](/sport/basketball/videos/cg640yk3n3zo)\\\\n\\\\n[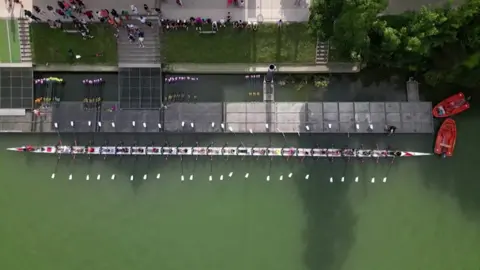\\\\\\\\\\\\n\\\\\\\\\\\\nWorld\\'s longest rowing boat to carry Olympic torch\\\\\\\\\\\\n--------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThe 24-seater boat will take the Olympic torch down a section of the River Marne on Sunday.\\\\\\\\\\\\n\\\\\\\\\\\\n1 day ago\\\\\\\\\\\\n\\\\\\\\\\\\nEurope](/news/videos/cqe6q917y1jo)\\\\n\\\\n[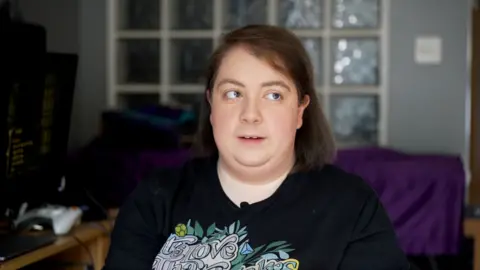\\\\\\\\\\\\n\\\\\\\\\\\\nAccessibility brings disabled gamers a sense of community\\\\\\\\\\\\n---------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nGreater accessibility in game development is opening the genre to more people with disabilities.\\\\\\\\\\\\n\\\\\\\\\\\\n1 day ago\\\\\\\\\\\\n\\\\\\\\\\\\nScotland](/news/videos/c0jq593xqk8o)\\\\n\\\\n[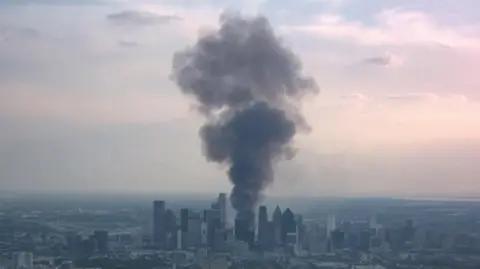\\\\\\\\\\\\n\\\\\\\\\\\\nWatch: Spire collapses as fire engulfs Texas church\\\\\\\\\\\\n---------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nA blaze at a historic church in Dallas has caused huge plumes of smoke to rise over the Texan city\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/videos/cjk325014g7o)\\\\n\\\\n* * *\\\\n\\\\nIn History\\\\n----------\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\n\\'He was after my life\\': WW1 soldier\\'s confession\\\\\\\\\\\\n------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nWorld War One broke out on 28 July, 1914. Fifty years later, one of the German soldiers, Stefan Westmann, told the BBC about his experiences fighting in the conflict.\\\\\\\\\\\\n\\\\\\\\\\\\nSee more](/culture/article/20240718-a-ww1-soldier-on-the-brutality-of-conflict)\\\\n\\\\n* * *\\\\n\\\\n[Olympic Games\\\\\\\\\\\\n-------------](https://www.bbc.com/sport/olympics)\\\\n\\\\u00a0\\\\n\\\\n[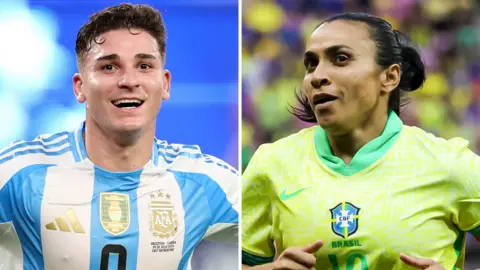\\\\\\\\\\\\n\\\\\\\\\\\\nTen footballers to watch out for at Paris Olympics\\\\\\\\\\\\n--------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nFrom Manchester City\\'s Julian Alvarez to Brazil icon Marta, BBC Sport picks out 10 footballers to watch at the Olympics.\\\\\\\\\\\\n\\\\\\\\\\\\nSee more](/sport/football/articles/cek91m98g48o)\\\\n\\\\n* * *\\\\n\\\\nUS and Canada news\\\\n------------------\\\\n\\\\n[\\'The right move but is it too late?\\' Democratic voters react\\\\\\\\\\\\n------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nJust now\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/crgk8rgm87lo)\\\\n\\\\n[Who could be Kamala Harris\\'s running mate?\\\\\\\\\\\\n------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n10 mins ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/c80ekdwk9zro)\\\\n\\\\n[Biden has backed Harris. What happens next in US election?\\\\\\\\\\\\n----------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n18 mins ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/articles/cq5xdq71drro)\\\\n\\\\n[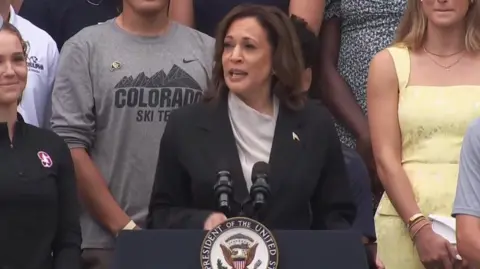\\\\\\\\\\\\n\\\\\\\\\\\\nBiden\\'s legacy of accomplishment is unmatched - Harris\\\\\\\\\\\\n------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThe US Vice-President praised Joe Biden\\'s track record as US president.\\\\\\\\\\\\n\\\\\\\\\\\\n34 mins ago\\\\\\\\\\\\n\\\\\\\\\\\\nUS & Canada](/news/videos/c51y936y9g2o)\\\\n\\\\n* * *\\\\n\\\\nGlobal news\\\\n-----------\\\\n\\\\n[At least six killed in Croatia nursing home shooting\\\\\\\\\\\\n----------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n7 mins ago\\\\\\\\\\\\n\\\\\\\\\\\\nEurope](/news/articles/cn08d7vyj6wo)\\\\n\\\\n[Israel orders evacuation of part of Gaza humanitarian zone\\\\\\\\\\\\n----------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nMiddle East](/news/articles/cgerz8we1vgo)\\\\n\\\\n[Russian-US journalist jailed for \\'false information\\'\\\\\\\\\\\\n----------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nEurope](/news/articles/cn08d7j1qj5o)\\\\n\\\\n[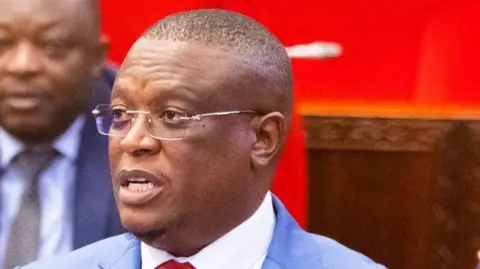\\\\\\\\\\\\n\\\\\\\\\\\\nTanzanian minister sacked after poll rigging remarks\\\\\\\\\\\\n----------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nNape Nnauye caused outrage for saying he could help an MP rig elections - comments, he said, he made in jest.\\\\\\\\\\\\n\\\\\\\\\\\\n3 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nAfrica](/news/articles/cd1e48677w0o)\\\\n\\\\n* * *\\\\n\\\\nUK news\\\\n-------\\\\n\\\\n[Campaigners in court over Magna Carta incident\\\\\\\\\\\\n----------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n54 mins ago\\\\\\\\\\\\n\\\\\\\\\\\\nUK](/news/articles/cxw29lg4y8go)\\\\n\\\\n[New Prince George photo released on 11th birthday\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n7 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUK](/news/articles/cw4yxd3dw7qo)\\\\n\\\\n[\\'We have one of the best comedy scenes in the UK\\'\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n12 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nUK](/news/articles/c4ngrdpwlx3o)\\\\n\\\\n[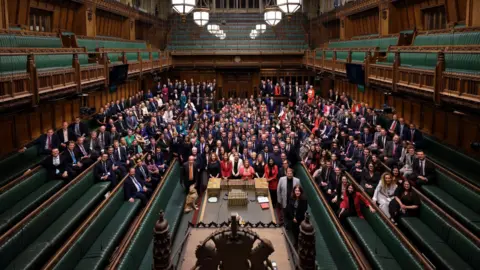\\\\\\\\\\\\n\\\\\\\\\\\\nHow does a surprise MP prepare for life in office?\\\\\\\\\\\\n--------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nNew MPs in the East describe the experience of unexpectedly picking up the reins of public life.\\\\\\\\\\\\n\\\\\\\\\\\\n12 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nEngland](/news/articles/cxr2g7rk3dxo)\\\\n\\\\n* * *\\\\n\\\\nSport\\\\n-----\\\\n\\\\n[Ferdinand\\'s persuasive powers sealed Man Utd\\'s Yoro deal\\\\\\\\\\\\n--------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n49 mins ago\\\\\\\\\\\\n\\\\\\\\\\\\nMan Utd](/sport/football/articles/c9e950ev0xpo)\\\\n\\\\n[Aston Villa complete \\\\u00a350m deal for Everton\\'s Onana\\\\\\\\\\\\n--------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nPremier League](/sport/football/articles/cgrlgzx6gpro)\\\\n\\\\n[Australia would not pick convicted rapist Olympian\\\\\\\\\\\\n--------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n3 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nOlympic Games](/sport/articles/c2v0j0j6nqlo)\\\\n\\\\n[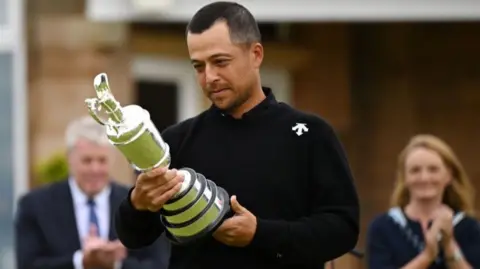\\\\\\\\\\\\n\\\\\\\\\\\\n\\'Schauffele passes ultimate examination in classic Open\\'\\\\\\\\\\\\n--------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThe 152nd Open should be remembered as a classic, writes BBC golf correspondent Iain Carter.\\\\\\\\\\\\n\\\\\\\\\\\\n1 hr ago\\\\\\\\\\\\n\\\\\\\\\\\\nGolf](/sport/golf/articles/cv2g1glwypqo)\\\\n\\\\n* * *\\\\n\\\\n[Video\\\\\\\\\\\\n-----](https://www.bbc.com/video)\\\\n\\\\u00a0\\\\n\\\\n[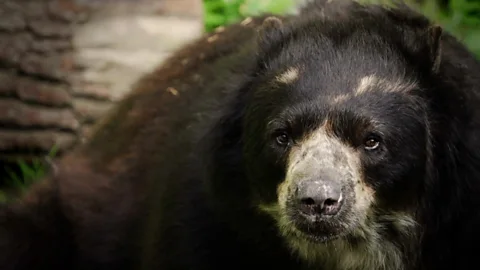\\\\\\\\\\\\n\\\\\\\\\\\\nThe \\'Paddington bears\\' facing climate threat\\\\\\\\\\\\n--------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nDrought forces the real Paddington Bear\\\\u00a0into deadly conflict with cattle farmers in the Andes.\\\\\\\\\\\\n\\\\\\\\\\\\nSee more](/reel/video/p0jbv222/climate-chaos-makes-paddington-bear-hangry-)\\\\n\\\\n* * *\\\\n\\\\nBusiness\\\\n--------\\\\n\\\\n[Ryanair set to slash summer fares as profits drop\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n8 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nBusiness](/news/articles/cj50d6q3jlro)\\\\n\\\\n[Former chancellor Zahawi mulling bid for the Telegraph\\\\\\\\\\\\n------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n26 mins ago\\\\\\\\\\\\n\\\\\\\\\\\\nBusiness](/news/articles/c4ng5q4jd62o)\\\\n\\\\n[Prime sued in trademark case by US Olympic committee\\\\\\\\\\\\n----------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n1 day ago](/news/articles/c4ng785gjv0o)\\\\n\\\\n[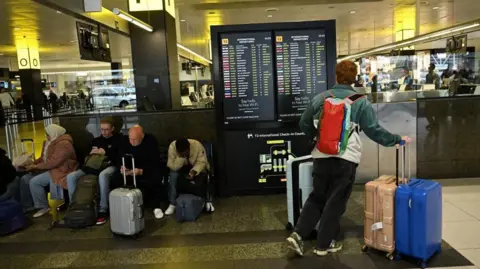\\\\\\\\\\\\n\\\\\\\\\\\\nCrowdStrike IT outage affected 8.5 million Windows devices, Microsoft says\\\\\\\\\\\\n--------------------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nIt\\\\u2019s the first time that a number has been put on the glitch that is still causing problems around the world.\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nTechnology](/news/articles/cpe3zgznwjno)\\\\n\\\\n* * *\\\\n\\\\nInnovation\\\\n----------\\\\n\\\\n[Company wins funding to make medicine in space\\\\\\\\\\\\n----------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n4 hrs ago](/news/articles/cp9vdxwjddeo)\\\\n\\\\n[Summer surge: why Covid-19 isn\\'t yet seasonal\\\\\\\\\\\\n---------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n1 day ago\\\\\\\\\\\\n\\\\\\\\\\\\nFuture](/future/article/20240719-why-covid-19-is-spreading-this-summer)\\\\n\\\\n[Scam warning as fake emails and websites target users after outage\\\\\\\\\\\\n------------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nTechnology](/news/articles/cq5xy12pynyo)\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\nAre fermented foods actually good for our health?\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nWhile humans have been eating fermented foods since ancient times, researchers are only starting to unravel some of the biggest questions about their health benefits.\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nFuture](/future/article/20240719-are-fermented-foods-actually-good-for-you)\\\\n\\\\n* * *\\\\n\\\\nCulture\\\\n-------\\\\n\\\\n[How to choose the most eco-friendly swimwear\\\\\\\\\\\\n--------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nCulture](/culture/article/20240719-how-to-choose-the-best-and-most-eco-friendly-swimwear)\\\\n\\\\n[In pictures: Colonial India through the eyes of foreign artists\\\\\\\\\\\\n---------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nAsia](/news/articles/c28ejl4nvgyo)\\\\n\\\\n[Auction for John Lennon glasses and Abbey Road photos\\\\\\\\\\\\n-----------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n1 day ago\\\\\\\\\\\\n\\\\\\\\\\\\nSurrey](/news/articles/cp085ym9l3ro)\\\\n\\\\n[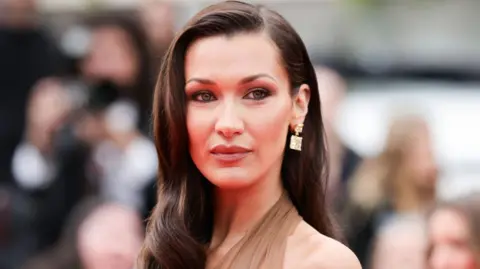\\\\\\\\\\\\n\\\\\\\\\\\\nBella Hadid\\'s Adidas advert dropped after Israeli criticism\\\\\\\\\\\\n-----------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThe model was chosen to promote shoes referencing the 1972 Munich Olympics, at which 11 Israeli athletes were killed.\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nCulture](/news/articles/ceqdwpv8vw1o)\\\\n\\\\n* * *\\\\n\\\\nTravel\\\\n------\\\\n\\\\n[The Indian dish Kamala Harris loves\\\\\\\\\\\\n-----------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n1 hr ago\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20201026-dosa-indias-wholesome-fast-food-obsession)\\\\n\\\\n[Italy\\'s most iconic trail reopens after 12 years\\\\\\\\\\\\n------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n3 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240722-cinque-terre-italys-path-of-love-reopens-after-12-years)\\\\n\\\\n[The US\\'s little-known \\'Ellis Island of the West\\'\\\\\\\\\\\\n------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n5 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240719-angel-island-the-little-known-ellis-island-of-the-west-us)\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\nThe Turkish ice cream eaten with a knife and fork\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nDondurma isn\\'t like any other ice cream you\\'ll find, and the epicentre of its production is still reeling from the powerful earthquakes that decimated the nation.\\\\\\\\\\\\n\\\\\\\\\\\\n1 day ago\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240719-dondurma-the-turkish-ice-cream-eaten-with-a-knife-and-fork)\\\\n\\\\n* * *\\\\n\\\\n[Travel\\\\\\\\\\\\n------](https://www.bbc.com/travel)\\\\n\\\\u00a0\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\nThe US\\'s little-known \\'Ellis Island of the West\\'\\\\\\\\\\\\n------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThis former quarantine and military station once processed as many as one million immigrants. Now, the picturesque island is one of the San Francisco Bay Area\\'s best urban getaways.\\\\\\\\\\\\n\\\\\\\\\\\\nSee more](/travel/article/20240719-angel-island-the-little-known-ellis-island-of-the-west-us)\\\\n\\\\n* * *\\\\n\\\\nSign up for our newsletters\\\\n---------------------------\\\\n\\\\n[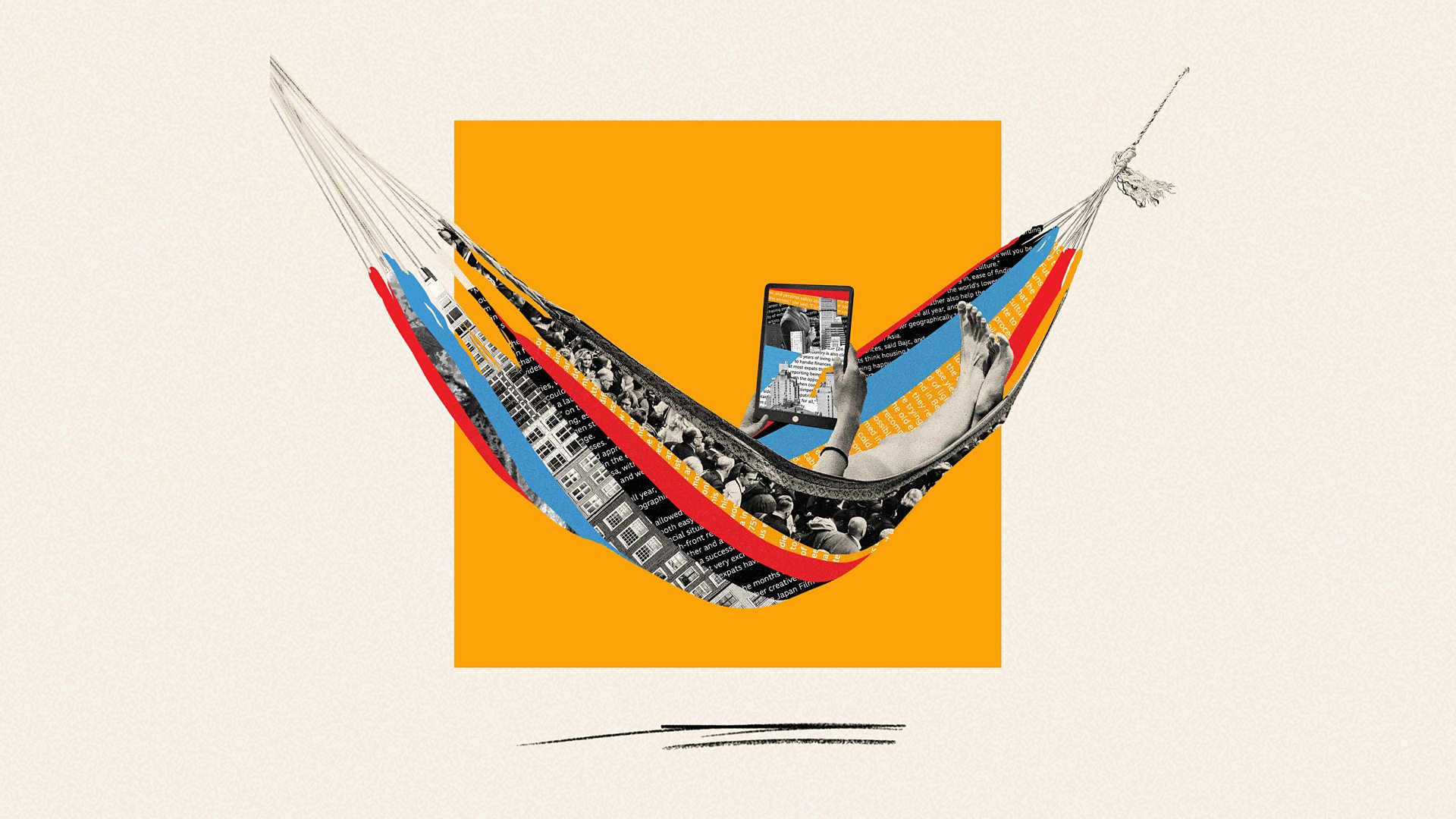\\\\\\\\\\\\n\\\\\\\\\\\\nThe Essential List\\\\\\\\\\\\n------------------\\\\\\\\\\\\n\\\\\\\\\\\\nThe week\\'s best stories, handpicked by BBC editors, in your inbox every Tuesday and Friday.](https://cloud.email.bbc.com/SignUp10_08?&at_bbc_team=studios&at_medium=display&at_objective=acquisition&at_ptr_type=&at_ptr_name=bbc.comhp&at_format=Module&at_link_origin=homepage&at_campaign=essentiallist&at_campaign_type=owned)\\\\n\\\\n[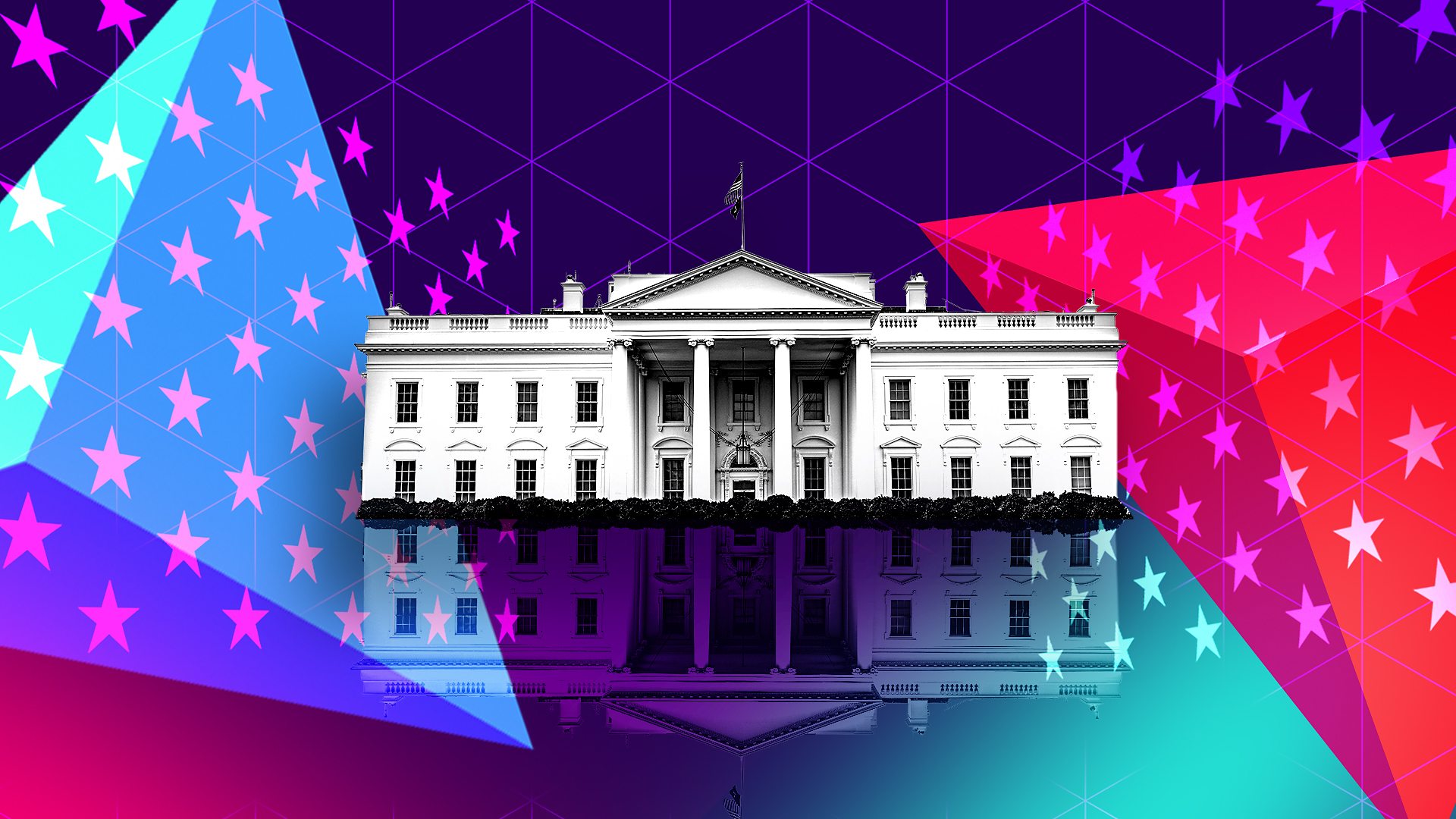\\\\\\\\\\\\n\\\\\\\\\\\\nUS Election Unspun\\\\\\\\\\\\n------------------\\\\\\\\\\\\n\\\\\\\\\\\\nCut through the spin with North America correspondent Anthony Zurcher - in your inbox every Wednesday.](https://cloud.email.bbc.com/US_Election_Unspun_newsletter_signup?&at_bbc_team=studios&at_medium=display&at_objective=acquisition&at_ptr_type=&at_ptr_name=bbc.comhp&at_format=Module&at_link_origin=homepage&at_campaign=uselectionunspun&at_campaign_type=owned)\\\\n\\\\n[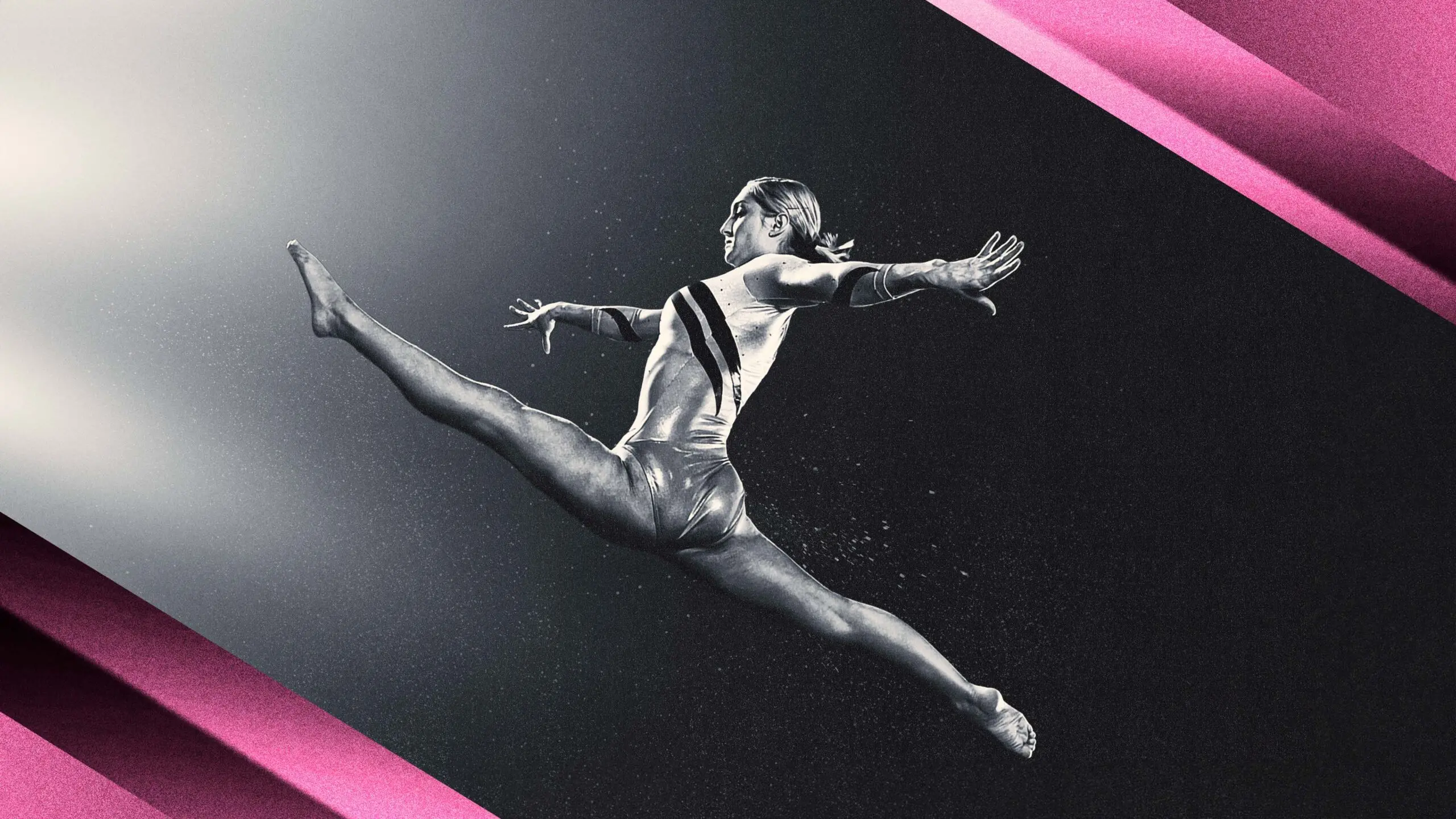\\\\\\\\\\\\n\\\\\\\\\\\\nMedal Moments\\\\\\\\\\\\n-------------\\\\\\\\\\\\n\\\\\\\\\\\\nYour global guide to the Paris Olympics, from key highlights to heroic stories, daily to your inbox throughout the Games.](https://cloud.email.bbc.com/medalmoments_newsletter_signup?&at_bbc_team=studios&at_medium=emails&at_objective=acquisition&at_ptr_type=&at_ptr_name=bbc.com&at_format=Module&at_link_origin=homepage&at_campaign=olympics2024&at_campaign_id=&at_adset_name=&at_adset_id=&at_creation=&at_creative_id=&at_campaign_type=owned)\\\\n\\\\n* * *\\\\n\\\\nEarth\\\\n-----\\\\n\\\\n[The \\'Paddington bears\\' facing climate threat\\\\\\\\\\\\n--------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nNatural wonders](/reel/video/p0jbv222/climate-chaos-makes-paddington-bear-hangry-)\\\\n\\\\n[The simple Japanese method for a tidier fridge\\\\\\\\\\\\n----------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n3 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nFuture](/future/article/20240715-the-simple-japanese-method-for-a-tidier-and-less-wasteful-fridge)\\\\n\\\\n[Conspiracy theories swirl about geo-engineering, but could it help save the planet?\\\\\\\\\\\\n-----------------------------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nBBC InDepth](/news/articles/c98qp79gj4no)\\\\n\\\\n[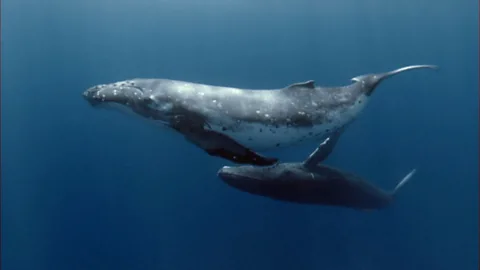\\\\\\\\\\\\n\\\\\\\\\\\\nFace to face with humpback whales\\\\\\\\\\\\n---------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nReece Parkinson discovers how locals are protecting their stunning marine environment.\\\\\\\\\\\\n\\\\\\\\\\\\n3 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/reel/video/p0jbpt60/face-to-face-with-humpback-whales)\\\\n\\\\n* * *\\\\n\\\\nScience and health\\\\n------------------\\\\n\\\\n[India alert after boy dies from Nipah virus in Kerala\\\\\\\\\\\\n-----------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n6 hrs ago\\\\\\\\\\\\n\\\\\\\\\\\\nAsia](/news/articles/cj50d7e9vp6o)\\\\n\\\\n[Are fermented foods actually good for our health?\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nFuture](/future/article/20240719-are-fermented-foods-actually-good-for-you)\\\\n\\\\n[Summer surge: why Covid-19 isn\\'t yet seasonal\\\\\\\\\\\\n---------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n1 day ago\\\\\\\\\\\\n\\\\\\\\\\\\nFuture](/future/article/20240719-why-covid-19-is-spreading-this-summer)\\\\n\\\\n[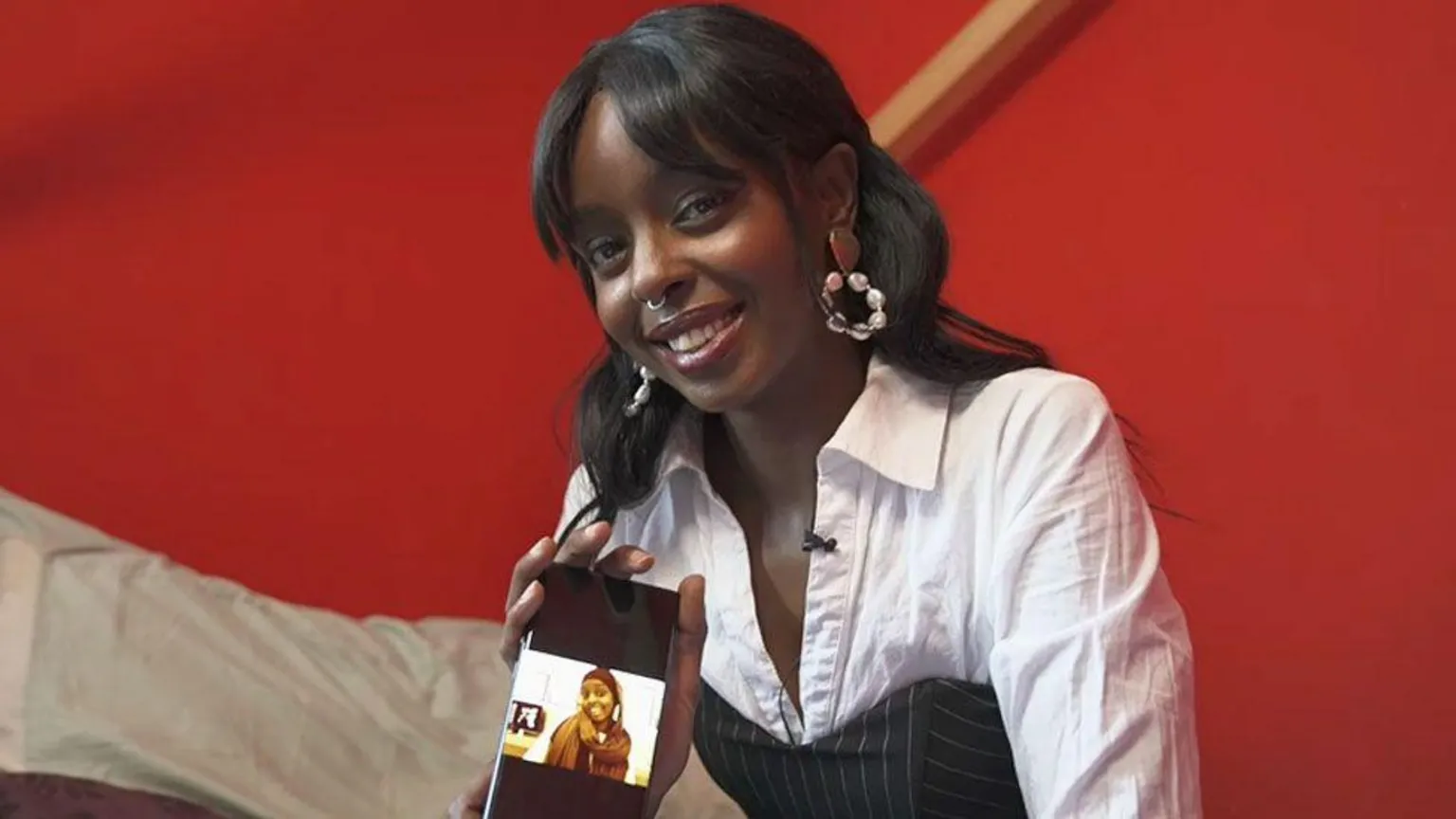\\\\\\\\\\\\n\\\\\\\\\\\\n\\'A way to fight back\\': FGM survivors reclaim vulvas with surgery\\\\\\\\\\\\n----------------------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nIn Somalia, it\\'s believed cutting off a girl\\'s outer genitalia will guarantee their virginity.\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nAfrica](/news/articles/cyx0perl8yno)\\\\n\\\\n* * *\\\\n\\\\nWorld\\\\u2019s Table\\\\n-------------\\\\n\\\\n[The Turkish ice cream eaten with a knife and fork\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n1 day ago\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240719-dondurma-the-turkish-ice-cream-eaten-with-a-knife-and-fork)\\\\n\\\\n[India\\'s cooling drinks to beat the heat\\\\\\\\\\\\n---------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n7 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240715-gond-katira-a-natural-way-to-cool-down-in-indias-scorching-summers)\\\\n\\\\n[The world\\'s biggest restaurant is coming to Paris\\\\\\\\\\\\n-------------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n9 Jul 2024\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240708-paris-olympics-2024-worlds-biggest-restaurant)\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\nThe wild ceremonies of the Turkish \\'meatball\\'\\\\\\\\\\\\n---------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nOne of the country\\'s most popular fast-food items, \\\\u00e7i\\\\u011f k\\\\u00f6fte is traditionally associated with wild and rowdy gatherings in south-eastern Turkey.\\\\\\\\\\\\n\\\\\\\\\\\\n1 Jun 2024\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240531-the-wild-ceremonies-surrounding-a-turkish-meatball)\\\\n\\\\n* * *\\\\n\\\\nThe Specialist\\\\n--------------\\\\n\\\\n[Guide to Helsinki\\'s happiest places\\\\\\\\\\\\n-----------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n2 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240718-a-happiness-hackers-guide-to-the-happiest-outdoor-places-in-helsinki)\\\\n\\\\n[A pastry chef\\'s favourite bakeries in Paris\\\\\\\\\\\\n-------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n5 days ago\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240717-david-lebovitzs-ultimate-guide-to-the-best-bakeries-in-paris-right-now)\\\\n\\\\n[Chef Andrew Zimmern\\'s favourite US restaurants\\\\\\\\\\\\n----------------------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\n14 Jul 2024\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240714-chef-andrew-zimmerns-favourite-us-restaurants)\\\\n\\\\n[\\\\\\\\\\\\n\\\\\\\\\\\\nA First Lady\\'s guide to Iceland\\\\\\\\\\\\n-------------------------------\\\\\\\\\\\\n\\\\\\\\\\\\nEliza Reid moved to Iceland 20 years ago for love and now she\\'s the First Lady. Here are her favourite ways to enjoy a \\\\\"chill\\\\\" Icelandic weekend.\\\\\\\\\\\\n\\\\\\\\\\\\n11 Jul 2024\\\\\\\\\\\\n\\\\\\\\\\\\nTravel](/travel/article/20240710-icelands-first-lady-takes-you-on-a-tour-of-her-super-chill-nation)\\\\n\\\\n* * *\\\\n\\\\n[British Broadcasting Corporation](/)\\\\n\\\\n* [Home](https://www.bbc.com/)\\\\n \\\\n* [News](/news)\\\\n \\\\n* [Sport](/sport)\\\\n \\\\n* [Business](/business)\\\\n \\\\n* [Innovation](/innovation)\\\\n \\\\n* [Culture](/culture)\\\\n \\\\n* [Travel](/travel)\\\\n \\\\n* [Earth](/future-planet)\\\\n \\\\n* [Video](/video)\\\\n \\\\n* [Live](/live)\\\\n \\\\n* [Audio](https://www.bbc.co.uk/sounds)\\\\n \\\\n* [Weather](https://www.bbc.com/weather)\\\\n \\\\n* [BBC Shop](https://shop.bbc.com/)\\\\n \\\\n\\\\nBBC in other languages\\\\n\\\\nFollow BBC on:\\\\n--------------\\\\n\\\\n* [Terms of Use](https://www.bbc.co.uk/usingthebbc/terms)\\\\n \\\\n* [About the BBC](https://www.bbc.co.uk/aboutthebbc)\\\\n \\\\n* [Privacy Policy](https://www.bbc.com/usingthebbc/privacy/)\\\\n \\\\n* [Cookies](https://www.bbc.com/usingthebbc/cookies/)\\\\n \\\\n* [Accessibility Help](https://www.bbc.co.uk/accessibility/)\\\\n \\\\n* [Contact the BBC](https://www.bbc.co.uk/contact)\\\\n \\\\n* [Advertise with us](https://www.bbc.com/advertisingcontact)\\\\n \\\\n* [Do not share or sell my info](https://www.bbc.com/usingthebbc/cookies/how-can-i-change-my-bbc-cookie-settings/)\\\\n \\\\n* [Contact technical support](https://www.bbc.com/contact-bbc-com-help)\\\\n \\\\n\\\\nCopyright 2024 BBC. All rights reserved.\\\\u00a0\\\\u00a0The _BBC_ is _not responsible for the content of external sites._\\\\u00a0[**Read about our approach to external linking.**](https://www.bbc.co.uk/editorialguidelines/guidance/feeds-and-links)\", \"html\": \"