diff --git a/README.md b/README.md
index 0d68af7..e4a2541 100644
--- a/README.md
+++ b/README.md
@@ -10,7 +10,7 @@ react-mobile-datepicker provides a component that can set year, month, day, hour
- is only 4k.
- It does not depend on moment.js
-## Theme
+## Theme
### default
@@ -35,22 +35,22 @@ react-mobile-datepicker provides a component that can set year, month, day, hour
### android-dark

-
-
-## Custom date unit
-
-set dateFormat for `['YYYY', 'MM', 'DD', 'hh', 'mm']` to configure year, month, day, hour, minute.
-
+
+
+## Custom date unit
+
+set dateFormat for `['YYYY', 'MM', 'DD', 'hh', 'mm']` to configure year, month, day, hour, minute.
+

-
-
-
-set dateFormat for `['hh', 'mm', 'ss']` to configure hour, minute and second.
-
+
+
+
+set dateFormat for `['hh', 'mm', 'ss']` to configure hour, minute and second.
+
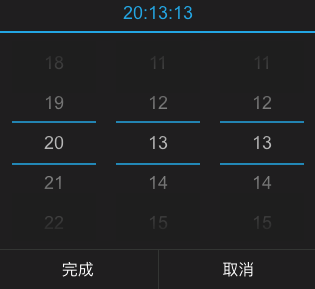
-
+
## Getting Started
@@ -122,17 +122,18 @@ ReactDOM.render(, document.getElementById('react-box'));
## PropTypes
| Property | Type | Default | Description |
-|:------------- |:------------- |:-------------- |:---------- |
+|:------------- |:------------- |:-------------- |:---------- |
| isPopup | Boolean | true | whether as popup add a overlay |
| isOpen | Boolean | false | whether to open datepicker |
| theme | String | default | theme of datepicker, include 'default', 'dark', 'ios', 'android', 'android-dark' |
-| dateFormat | Array | ['YYYY', 'M', 'D'] | according to year, month, day, hour, minute, second format specified display text. E.g ['YYYY年', 'MM月', 'DD日']|
+| dateFormat | Array | ['YYYY', 'M', 'D'] | according to year, month, day, hour, minute, second format specified display text. E.g ['YYYY年', 'MM月', 'DD日']|
+| dateSteps | Array | [1, 1, 1] | set step for each time unit |
|showFormat | String | 'YYYY/MM/DD' | customize the format of the display title |
| value | Date | new Date() | date value |
| min | Date | new Date(1970, 0, 1) | minimum date |
-| max | Date | new Date(2050, 0, 1) | maximum date |
-| showHeader | Boolean | true | whether to show the header |
-| customHeader | ReactElement | undefined | customize the header, if you set this property, it will replace `showFormat`|
+| max | Date | new Date(2050, 0, 1) | maximum date |
+| showHeader | Boolean | true | whether to show the header |
+| customHeader | ReactElement | undefined | customize the header, if you set this property, it will replace `showFormat`|
| confirmText | String | 完成 | customize the selection time button text |
| cancelText | String | 取消 | customize the cancel button text |
| onSelect | Function | () => {} | the callback function after click button of done, Date object as a parameter |
diff --git a/examples/basic/index.js b/examples/basic/index.js
index e6f8ed6..13c1620 100644
--- a/examples/basic/index.js
+++ b/examples/basic/index.js
@@ -7,7 +7,7 @@ import DatePicker from '../../lib/index';
(function main() {
class App extends React.Component {
state = {
- time: new Date(),
+ time: new Date(2016, 8, 16, 8, 20, 57),
isOpen: false,
theme: 'default',
}
@@ -59,8 +59,8 @@ import DatePicker from '../../lib/index';
{
expect(datePickerItems.at(2).props().format).to.equals('ss');
});
});
+
+describe('测试dateSteps属性', () => {
+ let props;
+ let mountedDatepicker;
+ let yearPicker, monthPicker, dayPicker;
+
+ const datePicker = () => {
+ if (!mountedDatepicker) {
+ mountedDatepicker = mount(
+
+ );
+
+ yearPicker = mountedDatepicker.find(DatePickerItem).first();
+ monthPicker = mountedDatepicker.find(DatePickerItem).at(1);
+ dayPicker = mountedDatepicker.find(DatePickerItem).at(2);
+ }
+
+ return mountedDatepicker;
+ }
+
+ beforeEach(() => {
+ props = {
+ value: new Date(2016, 8, 16, 8, 22, 57),
+ min: new Date(2015, 10, 1),
+ max: new Date(2020, 10, 1),
+ isOpen: true,
+ };
+ mountedDatepicker = undefined;
+ yearPicker = null;
+ monthPicker = null;
+ dayPicker = null;
+ });
+
+
+ it ('当datesteps等于[5, 5, 5], dateFormart等于[hh, mm, ss], 当前时间为8:20:57,向上滑动秒,分钟应该为23', () => {
+ props.dateFormat = ['hh', 'mm', 'ss'];
+ props.dateSteps = [1, 1, 5];
+
+ const datePickerItems = datePicker().find(DatePickerItem);
+ const second = dayPicker.find('.datepicker-viewport').instance();
+
+ const touchstartEvent = {
+ targetTouches: [{ pageY: 0 }],
+ };
+ const touchmoveEvent = {
+ targetTouches: [{ pageY: -21 }],
+ };
+ const touchendEvent = {
+ changedTouches: [{ pageY: -21 }],
+ };
+
+ eventTrigger(second, 'touchstart', touchstartEvent);
+ eventTrigger(second, 'touchmove', touchmoveEvent);
+ eventTrigger(second, 'touchend', touchendEvent);
+
+ return delay(250)
+ .then(() => {
+ expect(mountedDatepicker.state('value').getTime()).to.equals(new Date(2016, 8, 16, 8, 23, 2).getTime());
+ })
+ });
+
+
+ it ('当datesteps等于[5, 5, 5], dateFormart等于[hh, mm, ss], 当前时间为8:20:57,向上滑动秒,最大时间是8:20:59, 分钟应该为22', () => {
+ props.dateFormat = ['hh', 'mm', 'ss'];
+ props.dateSteps = [1, 1, 5];
+ props.max = new Date(2016, 8, 16, 8, 22, 59);
+
+ const datePickerItems = datePicker().find(DatePickerItem);
+ const second = dayPicker.find('.datepicker-viewport').instance();
+
+ const touchstartEvent = {
+ targetTouches: [{ pageY: 0 }],
+ };
+ const touchmoveEvent = {
+ targetTouches: [{ pageY: -21 }],
+ };
+ const touchendEvent = {
+ changedTouches: [{ pageY: -21 }],
+ };
+
+ eventTrigger(second, 'touchstart', touchstartEvent);
+ eventTrigger(second, 'touchmove', touchmoveEvent);
+ eventTrigger(second, 'touchend', touchendEvent);
+
+ return delay(250)
+ .then(() => {
+ expect(mountedDatepicker.state('value').getTime()).to.equals(new Date(2016, 8, 16, 8, 22, 57).getTime());
+ })
+ });
+});