diff --git a/.github/caption.png b/.github/caption.png
new file mode 100644
index 0000000..2e83717
Binary files /dev/null and b/.github/caption.png differ
diff --git a/README.md b/README.md
index 14489d8..68c917c 100644
--- a/README.md
+++ b/README.md
@@ -39,14 +39,69 @@ react-mobile-datepicker provides a component that can set year, month, day, hour
## Custom date unit
-set dateFormat for `['YYYY', 'MM', 'DD', 'hh', 'mm']` to configure year, month, day, hour, minute.
+set `dateConfig` to configure year, month, day, hour, minute.
+
+```javascript
+{
+ 'year': {
+ format: 'YYYY',
+ caption: 'Year',
+ step: 1,
+ },
+ 'month': {
+ format: 'MM',
+ caption: 'Mon',
+ step: 1,
+ },
+ 'date': {
+ format: 'DD',
+ caption: 'Day',
+ step: 1,
+ },
+ 'hour': {
+ format: 'hh',
+ caption: 'Hour',
+ step: 1,
+ },
+ 'minute': {
+ format: 'mm',
+ caption: 'Min',
+ step: 1,
+ },
+ 'second': {
+ format: 'hh',
+ caption: 'Sec',
+ step: 1,
+ },
+}
+```
-set dateFormat for `['hh', 'mm', 'ss']` to configure hour, minute and second.
+set `dateConfig` to configure hour, minute and second.
+
+```javascript
+{
+ 'hour': {
+ format: 'hh',
+ caption: 'Hour',
+ step: 1,
+ },
+ 'minute': {
+ format: 'mm',
+ caption: 'Min',
+ step: 1,
+ },
+ 'second': {
+ format: 'hh',
+ caption: 'Sec',
+ step: 1,
+ },
+}
+```
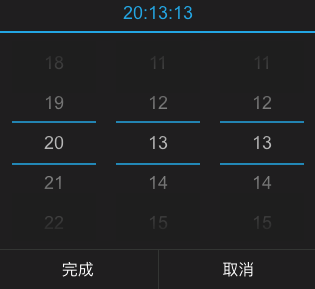
@@ -54,31 +109,80 @@ set dateFormat for `['hh', 'mm', 'ss']` to configure hour, minute and second.
customize the content mapping shown in the month.
-```js
+```javascript
+
const monthMap = {
- '01': 'Jan',
- '02': 'Feb',
- '03': 'Mar',
- '04': 'Apr',
- '05': 'May',
- '06': 'Jun',
- '07': 'Jul',
- '08': 'Aug',
- '09': 'Sep',
+ '1': 'Jan',
+ '2': 'Feb',
+ '3': 'Mar',
+ '4': 'Apr',
+ '5': 'May',
+ '6': 'Jun',
+ '7': 'Jul',
+ '8': 'Aug',
+ '9': 'Sep',
'10': 'Oct',
'11': 'Nov',
'12': 'Dec',
};
+const dateConfig = {
+ 'year': {
+ format: 'YYYY',
+ caption: 'Year',
+ step: 1,
+ },
+ 'month': {
+ format: value => monthMap[value.getMonth() + 1],
+ caption: 'Mon',
+ step: 1,
+ },
+ 'date': {
+ format: 'DD',
+ caption: 'Day',
+ step: 1,
+ },
+};
+
monthMap[month]], 'DD']}
+ dateConfig={dateConfig}
/>
```
-
+
+set `showCaption` to display date captions, matches the dateConfig property's caption.
+
+```javascript
+const dateConfig = {
+ 'hour': {
+ format: 'hh',
+ caption: 'Hour',
+ step: 1,
+ },
+ 'minute': {
+ format: 'mm',
+ caption: 'Min',
+ step: 1,
+ },
+ 'second': {
+ format: 'hh',
+ caption: 'Sec',
+ step: 1,
+ },
+}
+
+
+```
+
+
+

+
## Getting Started
@@ -93,7 +197,7 @@ Using [npm](https://www.npmjs.com/):
The following guide assumes you have some sort of ES2015 build set up using babel and/or webpack/browserify/gulp/grunt/etc.
-```js
+```javascript
// Using an ES6 transpiler like Babel
import React from 'react';
import ReactDOM from 'react-dom';
@@ -104,7 +208,7 @@ import DatePicker from 'react-mobile-datepicker';
### Usage Example
-```js
+```javascript
class App extends React.Component {
state = {
time: new Date(),
@@ -154,9 +258,11 @@ ReactDOM.render(, document.getElementById('react-box'));
| isPopup | Boolean | true | whether as popup add a overlay |
| isOpen | Boolean | false | whether to open datepicker |
| theme | String | default | theme of datepicker, include 'default', 'dark', 'ios', 'android', 'android-dark' |
-| dateFormat | Array | ['YYYY', 'M', 'D'] | according to year, month, day, hour, minute, second format specified display text. E.g ['YYYY年', 'MM月', 'DD日']|
-| dateSteps | Array | [1, 1, 1] | set step for each time unit |
-|showFormat | String | 'YYYY/MM/DD' | customize the format of the display title |
+| ~~dateFormat~~(deprecated, use `dateConfig` instead) | Array | ['YYYY', 'M', 'D'] | according to year, month, day, hour, minute, second format specified display text. E.g ['YYYY年', 'MM月', 'DD日']|
+| ~~dateSteps~~(deprecated), use `dateConfig` instead | Array | [1, 1, 1] | set step for each time unit |
+| dateConfig | Object | [See `DateConfig` format for details](#dateconfig) | configure date unit information |
+|~~showFormat~~(deprecated, use `headerFormat` instead) | String | 'YYYY/MM/DD' | customize the format of the display title |
+|headerFormat | String | 'YYYY/MM/DD' | customize the format of the display title |
| value | Date | new Date() | date value |
| min | Date | new Date(1970, 0, 1) | minimum date |
| max | Date | new Date(2050, 0, 1) | maximum date |
@@ -167,6 +273,52 @@ ReactDOM.render(, document.getElementById('react-box'));
| onSelect | Function | () => {} | the callback function after click button of done, Date object as a parameter |
| onCancel | Function | () => {} | the callback function after click button of cancel |
+
+## DateConfig
+
+all default date configuration information, as follows
+
+- format: date unit display format
+- caption: date unit caption
+- step: date unit change interval
+
+```javascript
+{
+ 'year': {
+ format: 'YYYY',
+ caption: 'Year',
+ step: 1,
+ },
+ 'month': {
+ format: 'M',
+ caption: 'Mon',
+ step: 1,
+ },
+ 'date': {
+ format: 'D',
+ caption: 'Day',
+ step: 1,
+ },
+ 'hour': {
+ format: 'hh',
+ caption: 'Hour',
+ step: 1,
+ },
+ 'minute': {
+ format: 'mm',
+ caption: 'Min',
+ step: 1,
+ },
+ 'second': {
+ format: 'hh',
+ caption: 'Sec',
+ step: 1,
+ },
+}
+
+```
+
+
## Changelog
* [Changelog](CHANGELOG.md)
diff --git a/lib/index.js b/lib/index.js
index b67cba2..38729e3 100644
--- a/lib/index.js
+++ b/lib/index.js
@@ -41,6 +41,7 @@ function ModalDatePicker({ isPopup, ...props }: ModalDatePickerProps) {
);
}
+ModalDatePicker.displayName = 'MobileDatePicker';
ModalDatePicker.defaultProps = defaultProps;
export default ModalDatePicker;
diff --git a/stories/index.css b/stories/index.css
new file mode 100644
index 0000000..97f4af1
--- /dev/null
+++ b/stories/index.css
@@ -0,0 +1,4 @@
+.datepicker {
+ width: 375px;
+ position: relative;
+}
\ No newline at end of file
diff --git a/stories/index.js b/stories/index.js
index 27aa361..d374c2e 100644
--- a/stories/index.js
+++ b/stories/index.js
@@ -5,6 +5,7 @@ import { storiesOf } from '@storybook/react';
// import { linkTo } from '@storybook/addon-links';
import DatePicker from '../lib/index.js';
+import './index.css';
const props = {
value: new Date(),
@@ -12,17 +13,9 @@ const props = {
theme: 'android'
};
-const wrapStyle = {
- width: 375,
- height: 294,
- position: 'relative',
-};
-
const getComponent = (options) => {
return (
-
-
-
+
);
};
@@ -34,11 +27,49 @@ storiesOf('Theme', module)
.addWithInfo('android', () => getComponent({theme: 'android'}))
.addWithInfo('android-dark', () => getComponent({theme: 'android-dark'}))
-storiesOf('dateFormat', module)
+
+ const dateConfigMap = {
+ 'year': {
+ format: 'YYYY',
+ caption: '年',
+ step: 1,
+ },
+ 'month': {
+ format: 'M',
+ caption: '月',
+ step: 1,
+ },
+ 'date': {
+ format: 'D',
+ caption: '日',
+ step: 1,
+ },
+ 'hour': {
+ format: 'hh',
+ caption: '时',
+ step: 1,
+ },
+ 'minute': {
+ format: 'mm',
+ caption: '分',
+ step: 1,
+ },
+ 'second': {
+ format: 'hh',
+ caption: '秒',
+ step: 1,
+ },
+};
+
+storiesOf('dateConfig', module)
.addWithInfo('YYYY,MM,DD', () => getComponent())
- .addWithInfo('YYYY,MM,DD hh:mm', () => getComponent({dateFormat: ['YYYY', 'MM', 'DD', 'hh', 'mm'], showFormat: 'YYYY/MM/DD hh:mm'}))
- .addWithInfo('hh:mm:ss', () => getComponent({dateFormat: ['hh', 'mm', 'ss'], showFormat: 'hh:mm:ss'}))
+ .addWithInfo('YYYY,MM,DD hh:mm', () => getComponent({dateConfig: dateConfigMap, showFormat: 'YYYY/MM/DD hh:mm'}))
+ .addWithInfo('hh:mm:ss', () => getComponent({dateConfig: ['hour', 'minute', 'second'], showFormat: 'hh:mm:ss'}))
storiesOf('dateLimit', module)
.addWithInfo('min', () => getComponent({ min: new Date(Date.now() - 3 * 24 * 60 * 60 * 1000) }))
.addWithInfo('max', () => getComponent({ max: new Date(Date.now() + 3 * 24 * 60 * 60 * 1000) }))
+
+storiesOf('dateCaption', module)
+ .addWithInfo('default caption', () => getComponent({dateConfig: ['year', 'month', 'date', 'hour', 'minute', 'second'], showCaption: true}))
+ .addWithInfo('custom caption', () => getComponent({dateConfig: dateConfigMap, showCaption: true}))
\ No newline at end of file