-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
2 changed files
with
223 additions
and
1 deletion.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,222 @@ | ||
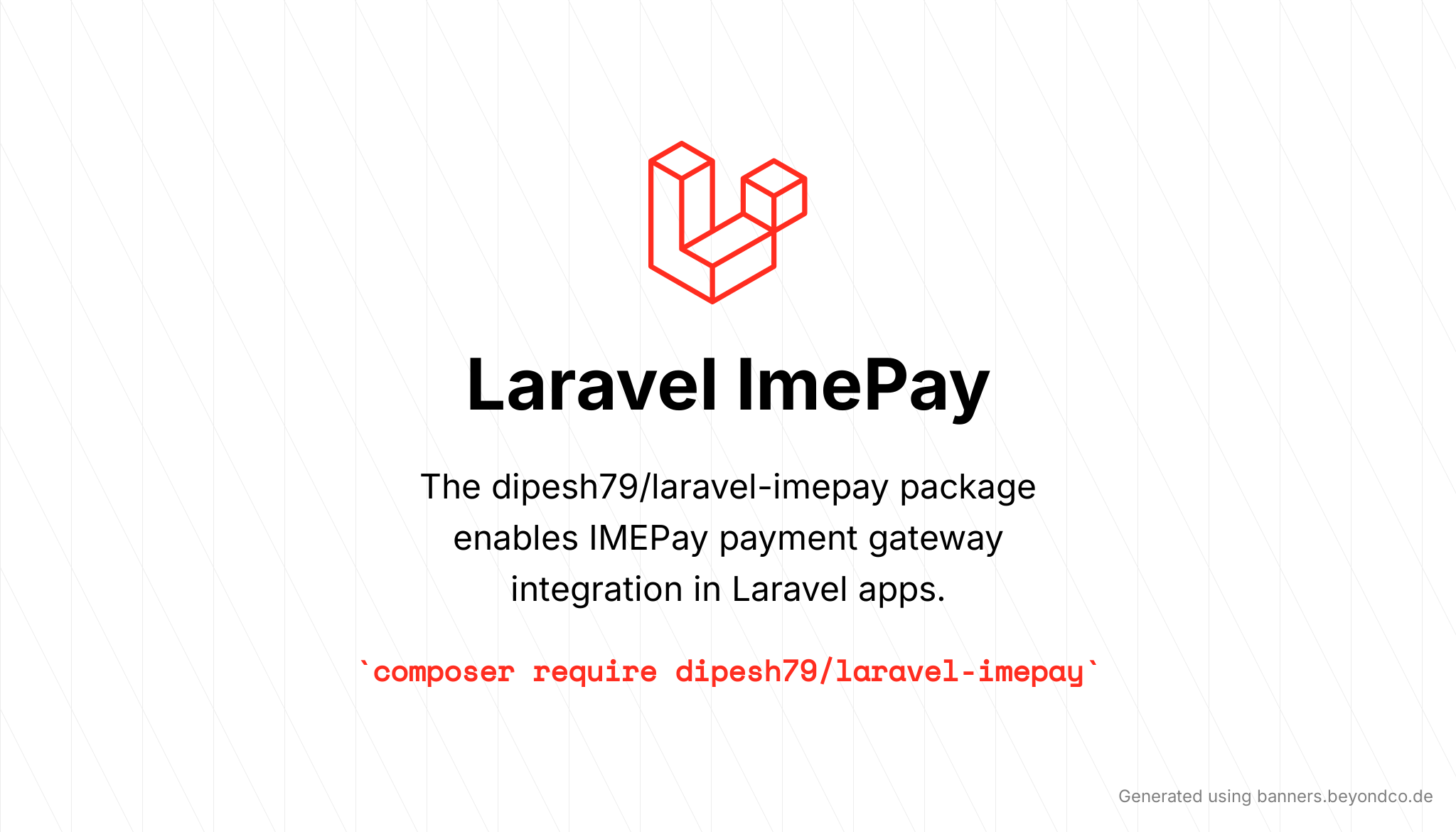 | ||
|
||
# Laravel ImePay | ||
|
||
[](https://packagist.org/packages/dipesh79/laravel-imepay) | ||
[](https://packagist.org/packages/dipesh79/laravel-imepay) | ||
[](https://packagist.org/packages/dipesh79/laravel-imepay) | ||
|
||
The `dipesh79/laravel-imepay` package enables IMEPay payment gateway integration in Laravel apps. | ||
|
||
## Installation | ||
|
||
Use composer to install the package. | ||
|
||
```bash | ||
composer require dipesh79/laravel-imepay | ||
``` | ||
|
||
## Usage | ||
|
||
### Add Variables in .env | ||
|
||
```bash | ||
IMEPAY_MERCHANT_CODE="IMEPAYTEST" | ||
IMEPAY_API_USER="imepaytest" | ||
IMEPAY_API_PASSWORD="imepaytest" | ||
IMEPAY_MODULE="API" | ||
IMEPAY_ENV="Sandbox" #Sandbox or Production | ||
IMEPAY_CALLBACK_URL="http://localhost:8000/imepay/callback" | ||
IMEPAY_CANCEL_URL="http://localhost:8000/imepay/cancel" | ||
IMEPAY_CALLBACK_METHOD="GET" | ||
|
||
``` | ||
|
||
### Publish Vendor File | ||
|
||
```bash | ||
php artisan vendor:publish | ||
``` | ||
|
||
And publish `Dipesh79\LaravelImePay\LaravelImePayServiceProvider` | ||
|
||
|
||
```php | ||
|
||
use Dipesh79\LaravelImePay\LaravelImepay; | ||
|
||
// create an instance | ||
$imepay = new LaravelImepay(); | ||
|
||
// generate a token | ||
$token = $imepay->generateToken($amount, $refId); | ||
|
||
// generate a checkout URL | ||
$url = $imepay->generateCheckOutUrl($token, $refId, $amount); | ||
|
||
// handle callback response | ||
$response = $imepay->callbackResponse($request); | ||
|
||
// confirm a payment | ||
$confirmation = $imepay->confirmPayment($refId, $tokenId, $transactionId, $msisdn); | ||
|
||
// recheck the status of a payment | ||
$status = $imepay->recheckPayment($refId, $tokenId); | ||
|
||
``` | ||
|
||
## Methods | ||
|
||
The `dipesh79/laravel-imepay` package provides the following methods to interact with the IMEPay API: | ||
|
||
1. **generateToken($amount, $refId)** | ||
- This method generates a token for the given amount and reference ID. | ||
- Parameters: | ||
- `$amount` - The amount to be paid. | ||
- `$refId` - The reference ID for the transaction. | ||
- Returns the token generated by the IMEPay API. | ||
- Throws an `ImePayException` if an error occurs during the operation. | ||
- Example: | ||
```php | ||
$token = $imepay->generateToken($amount, $refId); | ||
``` | ||
2. **generateCheckoutUrl($token, $refId, $amount)** | ||
|
||
- This method generates a checkout URL for the given token, reference ID, and amount. | ||
- Parameters: | ||
- `$token` - The token generated by the IMEPay API. | ||
- `$refId` - The reference ID for the transaction. | ||
- `$amount` - The amount to be paid. | ||
- Returns the checkout URL generated by the IMEPay API. | ||
- Throws an `ImePayException` if an error occurs during the operation. | ||
- Example: | ||
```php | ||
$url = $imepay->generateCheckoutUrl($token, $refId, $amount); | ||
``` | ||
|
||
3. **callbackResponse($request)** | ||
|
||
- This method handles the callback response from the IMEPay API. | ||
- Parameters: | ||
- `$request` - The request object containing the callback data. | ||
- Returns the response data from the IMEPay API. | ||
- Throws an `ImePayException` if an error occurs during the operation. | ||
- Example: | ||
```php | ||
$response = $imepay->callbackResponse($request); | ||
``` | ||
|
||
|
||
4. **confirmPayment($refId, $tokenId, $transactionId, $msisdn)** | ||
- This method confirms a payment with the given reference ID, token ID, transaction ID, and MSISDN. | ||
- Parameters: | ||
- `$refId` - The reference ID for the transaction. | ||
- `$tokenId` - The token ID generated by the IMEPay API. | ||
- `$transactionId` - The transaction ID returned by the IMEPay API. | ||
- `$msisdn` - The MSISDN of the user. | ||
- Returns the confirmation data from the IMEPay API. | ||
- Throws an `ImePayException` if an error occurs during the operation. | ||
- Example: | ||
```php | ||
$confirmation = $imepay->confirmPayment($refId, $tokenId, $transactionId, $msisdn); | ||
``` | ||
|
||
5. **recheckPayment($refId, $tokenId)** | ||
- This method rechecks the status of a payment with the given reference ID and token ID. | ||
- Parameters: | ||
- `$refId` - The reference ID for the transaction. | ||
- `$tokenId` - The token ID generated by the IMEPay API. | ||
- Returns the status data from the IMEPay API. | ||
- Throws an `ImePayException` if an error occurs during the operation. | ||
- Example: | ||
```php | ||
$status = $imepay->recheckPayment($refId, $tokenId); | ||
``` | ||
|
||
## Exceptions | ||
|
||
The `dipesh79/laravel-imepay` package throws specific exceptions to indicate problems that may occur during the execution of the IMEPay operations. Here are some of the exceptions and what they mean: | ||
|
||
1. **ImePayException** | ||
- This exception is thrown when an error occurs during the execution of an IMEPay operation. The exception message will contain the error message returned by the IMEPay API. | ||
- You can catch this exception and handle the error accordingly in your code. | ||
- Example: | ||
```php | ||
try { | ||
$token = $imepay->generateToken($amount, $refId); | ||
} catch (ImePayException $e) { | ||
// handle the error | ||
echo $e->getMessage(); | ||
} | ||
``` | ||
2. **InvalidKeyException** | ||
- This exception is thrown when the env key is missing. | ||
|
||
## Example | ||
|
||
Here is an example | ||
of how you can use the `dipesh79/laravel-imepay` package | ||
to integrate IMEPay payment gateway in your Laravel application: | ||
|
||
```php | ||
// Creating a Checkout page | ||
public function checkout(Request $request) | ||
{ | ||
$imepay = new \Dipesh79\LaravelImePay\LaravelImepay(); | ||
//Store this in Database for future reference. This is the unique reference ID for the transaction from your application | ||
$refId = uniqid(); | ||
$amount = 10; | ||
// Generating a Token | ||
$token = $imepay->generateToken($amount, $refId); | ||
//Store Token in Database for future reference | ||
// Generating a Checkout URL | ||
$url = $imepay->generateCheckOutUrl($token, $refId, $amount); | ||
// Redirecting the user to the IMEPay Checkout Page | ||
return redirect($url); | ||
} | ||
|
||
// Handling the Callback Response | ||
public function callback(Request $request) | ||
{ | ||
// Handling the Callback | ||
$imepay = new \Dipesh79\LaravelImePay\LaravelImepay(); | ||
$data = $imepay->callbackResponse($request); | ||
//Now check the status of the payment and update the database accordingly | ||
} | ||
|
||
public function confirmPayment(Request $request) | ||
{ | ||
$imepay = new \Dipesh79\LaravelImePay\LaravelImepay(); | ||
$refId = $request->refId; | ||
$tokenId = $request->tokenId; | ||
$transactionId = $request->transactionId; | ||
$msisdn = $request->msisdn; | ||
$confirmation = $imepay->confirmPayment($refId, $tokenId, $transactionId, $msisdn); | ||
//Now check the status of the payment and update the database accordingly | ||
} | ||
|
||
public function recheckPayment(Request $request) | ||
{ | ||
$imepay = new \Dipesh79\LaravelImePay\LaravelImepay(); | ||
$refId = $request->refId; | ||
$tokenId = $request->tokenId; | ||
$status = $imepay->recheckPayment($refId, $tokenId); | ||
//Now check the status of the payment and update the database accordingly | ||
} | ||
|
||
``` | ||
|
||
|
||
|
||
|
||
## License | ||
|
||
[MIT](https://choosealicense.com/licenses/mit/) | ||
|
||
## Author | ||
|
||
- [@Dipesh79](https://www.github.com/Dipesh79) | ||
|
||
## Support | ||
|
||
For support, email [dipeshkhanal79[at]gmail[dot]com](mailto:[email protected]). |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters