-
Notifications
You must be signed in to change notification settings - Fork 1
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Merge pull request #3 from DiegoYungh/master
add Iterator Behavioral pattern
- Loading branch information
Showing
17 changed files
with
144 additions
and
14 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,5 @@ | ||
import {Iterator} from './IteratorInterface'; | ||
|
||
export interface Container <T> { | ||
getIterator(): Iterator<T>; | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,30 @@ | ||
import {Iterator} from './IteratorInterface'; | ||
|
||
export class DemoIterator<T> implements Iterator<T> { | ||
private index: number; | ||
private items: Array<T>; | ||
|
||
constructor(items: Array<T>) { | ||
this.items = items; | ||
this.index = 0; | ||
} | ||
|
||
public currentItem() { | ||
return this.items[this.index]; | ||
} | ||
|
||
public hasNext() { | ||
return this.index < this.items.length - 1; | ||
} | ||
|
||
public next() { | ||
let item: any = null; | ||
|
||
if (this.hasNext() === true) { | ||
this.index = this.index + 1; | ||
item = this.items[this.index]; | ||
} | ||
|
||
return item; | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,14 @@ | ||
import {Container} from './ContainerInterface'; | ||
import {DemoIterator} from './DemoIterator'; | ||
|
||
export class DemoRepository<T> implements Container<T> { | ||
private items: Array<T>; | ||
|
||
public constructor(items: Array<T>) { | ||
this.items = items; | ||
} | ||
|
||
public getIterator() { | ||
return new DemoIterator(this.items); | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,5 @@ | ||
export interface Iterator<T> { | ||
currentItem(): T; | ||
hasNext(): boolean; | ||
next(): T; | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,32 @@ | ||
import { | ||
describe, | ||
it, | ||
expect, | ||
} from 'angular2/testing'; | ||
import {DemoRepository} from './DemoRepository'; | ||
|
||
export function main() { | ||
describe('Iterator', () => { | ||
|
||
let repository: DemoRepository<number> = new DemoRepository<number>( | ||
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10] | ||
); | ||
let iterator = repository.getIterator(); | ||
|
||
it('Should start on first', () => { | ||
let result = iterator.currentItem(); | ||
|
||
expect(result).toEqual(1); | ||
}); | ||
|
||
it('Should finish on last', () => { | ||
while (iterator.hasNext() !== false) { | ||
iterator.next(); | ||
} | ||
|
||
let result = iterator.currentItem(); | ||
|
||
expect(result).toEqual(10); | ||
}); | ||
}); | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,38 @@ | ||
`Iterator` | ||
========= | ||
|
||
Description: | ||
------------ | ||
|
||
Iterator pattern is very commonly used design pattern in Java and .Net programming environment. This pattern is used to get a way to access the elements of a collection object in sequential manner without any need to know its underlying representation. | ||
|
||
Iterator pattern falls under behavioral pattern category. | ||
|
||
Iterator example | ||
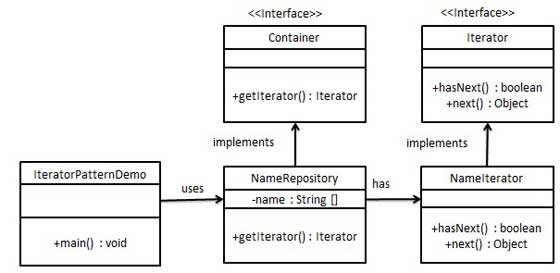 | ||
|
||
Implementation: | ||
--------------- | ||
|
||
We're going to create a Iterator interface which narrates navigation method and a Container interface which retruns the iterator . Concrete classes implementing the Container interface will be responsible to implement Iterator interface and use it | ||
|
||
IteratorPatternDemo, our demo class will use NamesRepository, a concrete class implementation to print a Names stored as a collection in NamesRepository. | ||
|
||
Files: | ||
------ | ||
|
||
[ContainerInterface](ContainerInterface.ts) | ||
|
||
[IteratorInterface](IteratorInterface.ts) | ||
|
||
[DemoIterator](DemoIterator.ts) | ||
|
||
[DemoRepository](DemoRepository.ts) | ||
|
||
[Demo](IteratorPatternDemo.spec.ts) | ||
|
||
## Running tests | ||
|
||
```bash | ||
npm test | ||
``` |